用Java的NIO技术写一个Knock Knock小游戏的客户机/服务器设计代码
时间: 2024-01-27 16:04:48 浏览: 140
好的,以下是一个基于Java NIO技术的Knock Knock小游戏的客户端/服务器端设计代码。
首先,我们需要定义一些常量和变量:
```java
public class KnockKnockServer {
private static final int PORT = 8080;
private Selector selector;
private ByteBuffer buffer = ByteBuffer.allocate(256);
private Map<SocketChannel, String> clientResponses = new HashMap<>();
private Map<SocketChannel, Integer> clientStates = new HashMap<>();
private Map<SocketChannel, Queue<String>> clientQueues = new HashMap<>();
private ServerSocketChannel serverChannel;
private int state = 0;
private String[] jokes = {"Knock, knock.", "Who’s there?", "Boo.", "Boo who?", "Don’t cry. It’s only a joke."};
}
```
然后,我们创建一个初始化方法,用于创建服务器端的SocketChannel和Selector对象:
```java
private void init() throws IOException {
selector = Selector.open();
serverChannel = ServerSocketChannel.open();
serverChannel.configureBlocking(false);
serverChannel.socket().bind(new InetSocketAddress(PORT));
serverChannel.register(selector, SelectionKey.OP_ACCEPT);
}
```
接下来,我们需要实现一个方法,用于处理客户端连接请求:
```java
private void accept(SelectionKey key) throws IOException {
SocketChannel client = serverChannel.accept();
client.configureBlocking(false);
client.register(selector, SelectionKey.OP_READ);
clientQueues.put(client, new LinkedList<>());
clientStates.put(client, 0);
System.out.println("Accepted new connection from " + client.getRemoteAddress());
}
```
然后,我们需要实现一个方法,用于处理客户端的读取请求:
```java
private void read(SelectionKey key) throws IOException {
SocketChannel client = (SocketChannel) key.channel();
buffer.clear();
int read = client.read(buffer);
if (read == -1) {
client.close();
return;
}
buffer.flip();
String clientResponse = new String(buffer.array(), 0, read);
System.out.println("Received: " + clientResponse + " from " + client.getRemoteAddress());
clientResponses.put(client, clientResponse);
processClientResponse(client);
key.interestOps(SelectionKey.OP_WRITE);
}
```
然后,我们需要实现一个方法,用于处理客户端的写入请求:
```java
private void write(SelectionKey key) throws IOException {
SocketChannel client = (SocketChannel) key.channel();
Queue<String> queue = clientQueues.get(client);
if (queue.isEmpty()) {
key.interestOps(SelectionKey.OP_READ);
return;
}
String message = queue.poll();
ByteBuffer buffer = ByteBuffer.wrap(message.getBytes());
client.write(buffer);
System.out.println("Sent: " + message + " to " + client.getRemoteAddress());
}
```
接下来,我们需要实现一个方法,用于处理客户端的响应:
```java
private void processClientResponse(SocketChannel client) throws IOException {
String response = clientResponses.get(client);
int currentState = clientStates.get(client);
switch (currentState) {
case 0:
if (!response.equals("Knock, knock.")) {
clientQueues.get(client).offer("You're supposed to say \"Knock, knock.\" Try again.\n");
} else {
clientStates.put(client, 1);
clientQueues.get(client).offer("Who's there?\n");
}
break;
case 1:
if (!response.equals("Boo.")) {
clientQueues.get(client).offer("You're supposed to say \"Boo.\" Try again.\n");
} else {
clientStates.put(client, 2);
clientQueues.get(client).offer("Boo who?\n");
}
break;
case 2:
if (!response.equals("Don't cry. It's only a joke.")) {
clientQueues.get(client).offer("You're supposed to say \"Don't cry. It's only a joke.\" Try again.\n");
} else {
clientStates.put(client, 0);
clientQueues.get(client).offer("Knock, knock.\n");
}
break;
}
}
```
最后,我们需要实现一个方法,用于运行服务器:
```java
public void run() throws IOException {
init();
while (true) {
selector.select();
Iterator<SelectionKey> keys = selector.selectedKeys().iterator();
while (keys.hasNext()) {
SelectionKey key = keys.next();
keys.remove();
if (!key.isValid()) {
continue;
}
if (key.isAcceptable()) {
accept(key);
} else if (key.isReadable()) {
read(key);
} else if (key.isWritable()) {
write(key);
}
}
}
}
```
以上就是基于Java NIO技术的Knock Knock小游戏的客户端/服务器端设计代码,希望对你有帮助!
阅读全文
相关推荐
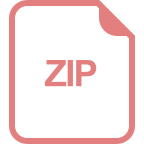
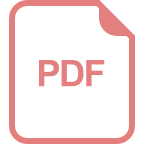


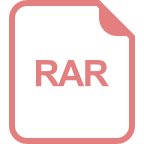
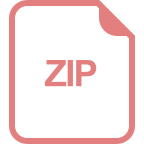
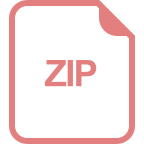
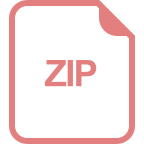
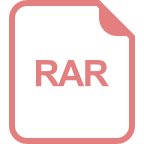
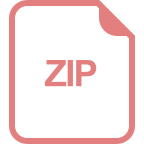
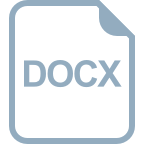
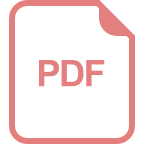
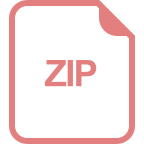