帮我用中文注释一下代码:// ThreadTester.java // Multiple threads printing at different intervals. public class ThreadTester { public static void main( String [] args ) { // create and name each thread PrintThread thread1 = new PrintThread( "thread1" ); PrintThread thread2 = new PrintThread( "thread2" ); PrintThread thread3 = new PrintThread( "thread3" ); System.err.println( "主线程将要启动三个线程" ); thread1.start(); // start thread1 and place it in ready state thread2.start(); // start thread2 and place it in ready state thread3.start(); // start thread3 and place it in ready state System.err.println( "三个线程启动完成, 主线程运行结束\n" ); } // end main } // end class ThreadTester // class PrintThread controls thread execution class PrintThread extends Thread { private int sleepTime; // assign name to thread by calling superclass constructor public PrintThread( String name ) { super( name ); // pick random sleep time between 0 and 5 seconds sleepTime = ( int ) ( Math.random() * 5001 ); } // method run is the code to be executed by new thread public void run() { // put thread to sleep for sleepTime amount of time try { System.err.println( getName() + " 进入睡眠状态,睡眠时间是: " + sleepTime ); Thread.sleep( sleepTime ); } // if thread interrupted during sleep, print stack trace catch ( InterruptedException exception ) { } // print thread name System.err.println( getName() + " 睡眠醒来" ); } // end method run } // end class PrintThread
时间: 2024-02-15 11:26:52 浏览: 24
// ThreadTester.java
// 多个线程在不同时间间隔打印。
public class ThreadTester {
public static void main(String[] args) {
// 创建并为每个线程命名
PrintThread thread1 = new PrintThread("线程1");
PrintThread thread2 = new PrintThread("线程2");
PrintThread thread3 = new PrintThread("线程3");
System.err.println("主线程将要启动三个线程");
// 启动线程1、2、3,并将它们放在就绪状态
thread1.start();
thread2.start();
thread3.start();
System.err.println("三个线程启动完成,主线程运行结束\n");
}
}
// 类PrintThread控制线程执行
class PrintThread extends Thread {
private int sleepTime; // 睡眠时间
// 调用父类构造函数为线程分配名称
public PrintThread(String name) {
super(name);
// 随机分配睡眠时间(0~5秒)
sleepTime = (int)(Math.random() * 5001);
}
// run方法是新线程要执行的代码
public void run() {
// 让线程睡眠sleepTime时间
try {
System.err.println(getName() + " 进入睡眠状态,睡眠时间是: " + sleepTime);
Thread.sleep(sleepTime);
}
// 如果在睡眠期间线程被中断,就打印堆栈跟踪信息
catch (InterruptedException exception) {}
// 打印线程名称
System.err.println(getName() + "睡眠醒来");
}
}
相关推荐
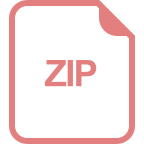
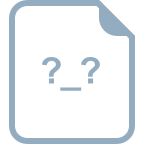
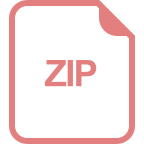














