在keil平台生成NRF52832芯片软件模拟IIC的代码
时间: 2024-06-05 08:06:32 浏览: 188
以下是在Keil平台生成NRF52832芯片软件模拟IIC的代码:
#include "nrf_delay.h"
#include "nrf_gpio.h"
// 定义IIC总线的GPIO引脚
#define SDA_PIN 17
#define SCL_PIN 16
// 定义读写标志位
#define IIC_READ 1
#define IIC_WRITE 0
// 定义IIC总线的速度
#define IIC_SPEED 100000
// 定义IIC总线上的从设备地址
#define IIC_ADDR 0x50
// 写IIC总线一个字节
void iic_write_byte(uint8_t data) {
uint8_t i;
for(i = 0; i < 8; i++) {
if(data & 0x80) {
nrf_gpio_pin_set(SDA_PIN);
} else {
nrf_gpio_pin_clear(SDA_PIN);
}
nrf_delay_us(1);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
nrf_gpio_pin_clear(SCL_PIN);
data <<= 1;
nrf_delay_us(1);
}
nrf_gpio_pin_set(SDA_PIN);
nrf_delay_us(1);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
nrf_gpio_pin_clear(SCL_PIN);
nrf_delay_us(1);
}
// 从IIC总线读一个字节
uint8_t iic_read_byte(uint8_t ack) {
uint8_t i;
uint8_t data = 0;
nrf_gpio_pin_set(SDA_PIN);
for(i = 0; i < 8; i++) {
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
data = (data << 1) | nrf_gpio_pin_read(SDA_PIN);
nrf_gpio_pin_clear(SCL_PIN);
nrf_delay_us(1);
}
if(ack) {
nrf_gpio_pin_clear(SDA_PIN);
} else {
nrf_gpio_pin_set(SDA_PIN);
}
nrf_delay_us(1);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
nrf_gpio_pin_clear(SCL_PIN);
nrf_delay_us(1);
nrf_gpio_pin_set(SDA_PIN);
nrf_delay_us(1);
return data;
}
// 初始化IIC总线
void iic_init(void) {
nrf_gpio_cfg_output(SDA_PIN);
nrf_gpio_cfg_output(SCL_PIN);
nrf_gpio_pin_set(SDA_PIN);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(5);
}
// 从IIC总线读取数据
void iic_read_data(uint8_t addr, uint8_t *data, uint8_t len) {
uint8_t i;
iic_init();
iic_write_byte((addr << 1) | IIC_READ);
for(i = 0; i < (len - 1); i++) {
data[i] = iic_read_byte(IIC_READ);
}
data[len - 1] = iic_read_byte(IIC_WRITE);
nrf_gpio_pin_set(SDA_PIN);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
}
// 向IIC总线写入数据
void iic_write_data(uint8_t addr, uint8_t *data, uint8_t len) {
uint8_t i;
iic_init();
iic_write_byte((addr << 1) | IIC_WRITE);
for(i = 0; i < len; i++) {
iic_write_byte(data[i]);
}
nrf_gpio_pin_set(SDA_PIN);
nrf_gpio_pin_set(SCL_PIN);
nrf_delay_us(1);
}
int main(void) {
uint8_t data[4] = {0x12, 0x34, 0x56, 0x78};
iic_write_data(IIC_ADDR, data, 4);
iic_read_data(IIC_ADDR, data, 4);
return 0;
}
阅读全文
相关推荐
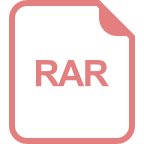


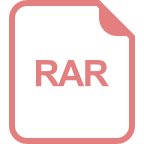
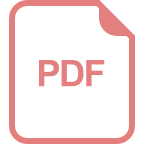
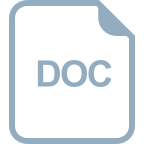
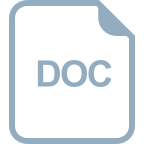
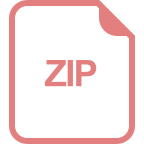
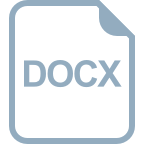
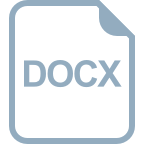