基于resnet图像目标分类项目
时间: 2023-12-06 08:01:03 浏览: 45
基于resnet图像目标分类项目,我们将使用深度学习的方法来对图像进行识别和分类。ResNet是一种非常有效的深度卷积神经网络结构,可以帮助我们在图像处理任务中取得良好的性能。在这个项目中,我们首先需要收集并准备好包含不同目标的图像数据集。然后,我们可以使用ResNet模型来对这些图像进行训练,以便模型能够学习到不同目标的特征和区分能力。
在训练过程中,我们需要将数据集分为训练集和测试集,确保模型在未见过的数据上也能有良好的泛化能力。我们还需要对输入图像进行预处理,包括大小调整、归一化等步骤,以便模型能更好地学习到图像中的特征。训练完成后,我们可以使用测试集来评估模型的性能,包括准确率、召回率等指标。
在项目中,我们还可以进行模型调优和迁移学习,以进一步提高模型的分类准确率。同时,我们还可以尝试不同的数据增强技术,如随机裁剪、旋转、翻转等,来增加数据集的多样性,进而改善模型的泛化能力。最终,我们可以将训练好的模型部署到实际应用中,用来对新的图像进行分类识别,例如人脸识别、物体识别等场景中。通过这样的项目,我们可以更好地理解和应用深度学习技术在图像处理领域的能力和潜力。
相关问题
基于resnet实现目标检测
基于ResNet实现目标检测是一种常见的方法,下面是一个简要的介绍:
ResNet(Residual Network)是一种深度卷积神经网络,通过引入残差连接(residual connection)解决了深层网络训练过程中的梯度消失和梯度爆炸问题。目标检测是计算机视觉领域的一个重要任务,旨在从图像中识别和定位特定目标。
基于ResNet的目标检测方法通常采用两个主要步骤:特征提取和目标定位。
1. 特征提取:首先,使用预训练的ResNet模型作为特征提取器。这意味着我们可以利用在大规模图像数据集上预训练好的ResNet模型来提取图像的高级特征。通过将图像输入到ResNet模型中,我们可以获取到图像在不同层级上的特征表示。
2. 目标定位:在特征提取的基础上,我们可以使用不同的目标检测算法来定位目标。常见的目标检测算法包括Faster R-CNN、YOLO(You Only Look Once)和SSD(Single Shot MultiBox Detector)等。这些算法通常使用卷积神经网络(CNN)来对提取的特征进行分类和定位。
在目标检测过程中,我们可以根据具体需求选择不同的ResNet模型,如ResNet-50、ResNet-101或ResNet-152等。这些模型的主要区别在于网络的深度和参数数量,更深的网络通常具有更强的特征提取能力,但也会增加计算复杂度。
基于ResNet18网络完成图像分类任务
好的,针对基于ResNet18网络完成图像分类任务,我可以给你一些基本的解释和思路,希望能够帮到你。
ResNet18是一种经典的卷积神经网络模型,它是由微软研究院提出的,被广泛应用于图像分类、目标检测等领域。图像分类任务是指将输入的图像分为预定义的类别中的一种,通常使用监督学习方法。我们可以使用ResNet18进行图像分类任务的实现,具体步骤如下:
1. 数据预处理:首先需要将图像数据进行预处理,如图像缩放、归一化等操作。
2. 模型搭建:使用PyTorch等深度学习框架,搭建ResNet18网络模型,包括卷积层、池化层、全连接层等。
3. 模型训练:将预处理后的图像数据输入到ResNet18网络模型中进行训练,使用交叉熵等损失函数进行优化。
4. 模型评估:使用测试集合评估模型的性能,包括准确率、召回率等指标。
5. 模型应用:将训练好的模型应用到实际任务中,进行图像分类。
总的来说,基于ResNet18网络完成图像分类任务的关键在于数据预处理、模型搭建和训练、模型评估和应用。希望这些思路能够帮到你。
相关推荐
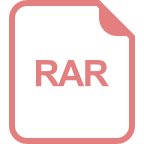
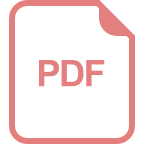
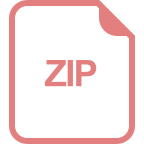
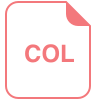
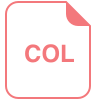
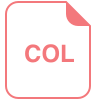
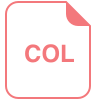
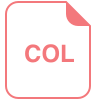







