如何在C语言中使用生产者/消费者模式构建一个包含两个线程(一个作为生产者,另一个作为消费者)的控制台程序,确保线程之间的同步和互斥操作得到正确实现?
时间: 2024-12-10 19:47:52 浏览: 10
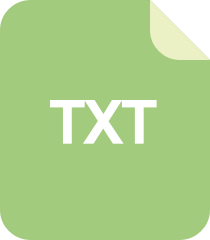
使用多线程程序模拟实现单生产者/多消费者问题(Linux下C语言)。
在C语言中,我们可以使用`pthread.h`库来创建和管理线程,并结合一些互斥锁(如`pthread_mutex_t`)和条件变量(如`pthread_cond_t`)来实现生产者/消费者模式。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_ITEMS 10
typedef struct {
int items[MAX_ITEMS];
int count;
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t cond; // 条件变量
} Queue;
void* producer(void* arg) {
Queue* queue = (Queue*)arg;
while (1) {
// 生产物品并获取锁
pthread_mutex_lock(&queue->mutex);
if (queue->count < MAX_ITEMS) {
queue->items[queue->count] = ++queue->count;
printf("Producer added item %d\n", queue->count);
} else {
printf("Producer: Buffer full, waiting...\n");
pthread_cond_wait(&queue->cond, &queue->mutex); // 等待
}
pthread_mutex_unlock(&queue->mutex);
}
return NULL;
}
void* consumer(void* arg) {
Queue* queue = (Queue*)arg;
while (1) {
// 消费物品并获取锁
pthread_mutex_lock(&queue->mutex);
if (queue->count > 0) {
int consumed = queue->items[0];
queue->items[0] = 0; // 清空位置
queue->count--;
printf("Consumer consumed item %d\n", consumed);
} else {
printf("Consumer: Buffer empty, waiting...\n");
pthread_cond_wait(&queue->cond, &queue->mutex); // 等待
}
pthread_mutex_unlock(&queue->mutex);
}
return NULL;
}
int main() {
Queue queue;
pthread_mutex_init(&queue.mutex, NULL);
pthread_cond_init(&queue.cond, NULL);
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, &queue);
pthread_create(&consumer_thread, NULL, consumer, &queue);
// 等待所有线程结束
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
pthread_mutex_destroy(&queue.mutex);
pthread_cond_destroy(&queue.cond);
return 0;
}
```
在这个例子中,生产者和消费者通过`mutex`互斥地访问队列,当缓冲区满时生产者等待,当缓冲区为空时消费者等待。`cond_wait`函数用于线程间通信,使得它们可以在满足特定条件后继续执行。
阅读全文
相关推荐
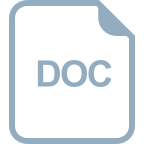
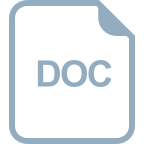
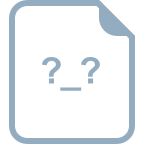
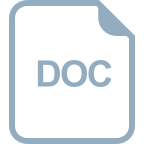
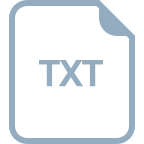
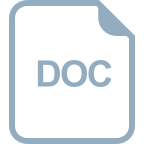
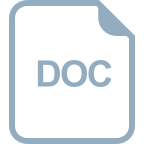
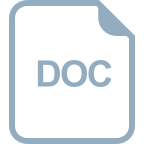
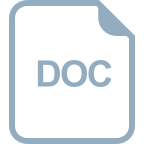








