Linux生产者/消费者问题C语言
时间: 2024-07-16 09:00:18 浏览: 90
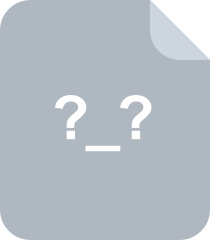
Linux c语言多线程实现生产者/消费者问题
Linux 生产者-消费者问题(Producer-Consumer Problem)是一个经典的并发编程示例,它涉及到两个线程角色:生产者和消费者,使用队列来管理数据交换。在C语言中,这个问题通常通过互斥锁(mutex)、条件变量(condition variables)或信号量(semaphores)来解决,以确保线程间的同步和避免数据竞争。
以下是基本的C语言实现步骤:
1. 创建一个共享的数据结构(如队列或环形缓冲区),用于存储生产者产生的数据。
2. 使用互斥锁保护数据结构,确保任何时候只有一个线程可以访问它。
3. **生产者**线程负责生成数据,并将数据放入数据结构。当队列满或者没有空闲位置时,生产者会进入等待状态,直到消费者处理了部分数据。
4. **消费者**线程负责从数据结构中取出数据并处理。当队列为空时,消费者会进入等待状态,直到生产者添加了新的数据。
5. 使用条件变量来触发线程从等待状态唤醒,只有满足特定条件(如队列非空或非满)时,线程才会被唤醒。
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
sem_t empty, full;
int buffer[BUFFER_SIZE];
int count = 0;
int in_prod = 0;
int in_cons = 0;
void *producer(void *arg) {
while (1) {
sem_wait(&empty); // 当队列满时等待
buffer[in_prod] = 'P'; // 生产数据
in_prod = (in_prod + 1) % BUFFER_SIZE;
count++; // 增加计数
sem_post(&full); // 队列不满时通知消费者
}
}
void *consumer(void *arg) {
while (1) {
sem_wait(&full); // 当队列空时等待
printf("Consuming: %c\n", buffer[in_cons]);
in_cons = (in_cons + 1) % BUFFER_SIZE;
count--; // 减少计数
sem_post(&empty); // 队列不空时通知生产者
}
}
int main() {
pthread_t producer_thread, consumer_thread;
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
阅读全文
相关推荐
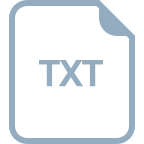
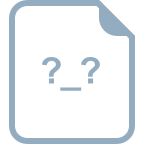
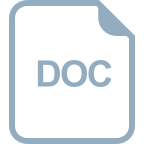
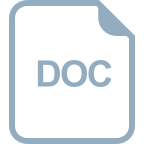
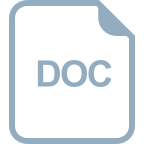
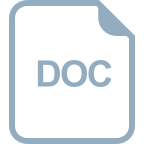
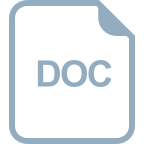
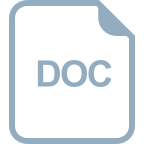
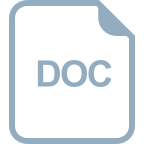
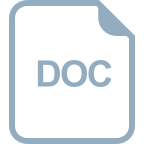
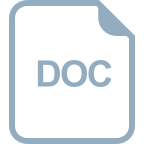
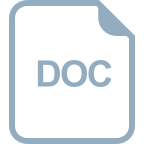
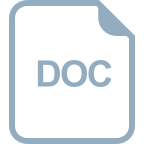
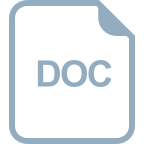
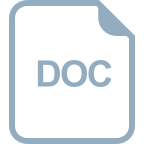

