c++如何利用ffmpeg更改MP4的视频时长
时间: 2024-10-06 07:01:17 浏览: 47
在C++中,你可以通过FFmpeg库来处理MP4视频并更改其时长。FFmpeg是一个功能强大的多媒体框架,包含了编码、解码、流处理等众多工具。以下是使用FFmpeg C++接口(如`libavformat`和`libavcodec`)来缩短MP4视频时长的基本步骤:
1. **安装FFmpeg**:首先确保你已经安装了FFmpeg,并且它的包含头文件和库路径添加到了你的C++项目的构建环境中。
2. **链接FFmpeg库**:在你的C++项目中,需要链接到`libavformat`和`libavcodec`库。
3. **创建AVFormatContext结构体**:这是FFmpeg的核心结构,用于表示媒体文件或流。
```cpp
#include <libavformat/avformat.h>
// 其他必要的FFmpeg头文件
AVFormatContext* pFormatCtx = NULL;
```
4. **打开输入文件**:
使用`avformat_open_input()`函数打开MP4文件。
```cpp
if (!avformat_open_input(&pFormatCtx, "input.mp4", NULL, NULL)) {
// 处理错误
}
```
5. **查找并注册编解码器**:
找到视频和音频编解码器,以便后续操作。
```cpp
av_register_all();
```
6. **解析输入格式**:
通过`avformat_find_stream_info()`解析输入文件的元数据。
```cpp
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
// 处理错误
}
```
7. **获取视频流**:
根据索引找到视频流,并保存其指针。
```cpp
AVCodecContext* videoCodecCtx = pFormatCtx->streams[videoStreamIndex]->codec;
```
8. **设置新的时长**:
创建一个新的时间戳列表,表示新时长。
```cpp
int newDuration = oldDuration * 新的比例; // 新的时长(秒)
AVRational timeBase = {1, AV_TIME_BASE};
AVTime duration = av_rescale_q(newDuration, &timeBase, videoCodecCtx->time_base);
```
9. **截取视频数据**:
使用`av_interleaved_write_frame()`从原始帧缓存中截取指定时长的数据。
```cpp
while (av_read_frame(pFormatCtx, &pkt) >= 0) {
if (pkt.stream_index == videoStreamIndex) {
int64_t startTs = pkt.dts + duration.num / duration.den;
int64_t endTs = startTs + duration.num / duration.den;
// 截取数据...
}
av_free_packet(&pkt);
}
// 当读取完成后关闭输入文件
avformat_close_input(&pFormatCtx);
```
10. **输出到新的MP4文件**:
如果你想保存修改后的视频,需要创建一个新的输出文件,然后将截取的数据写入其中,最后关闭输出文件。
注意:这只是一个基本的示例,实际操作中可能还需要处理错误检查、编码格式转换等问题。完整的C++代码通常会封装在循环或回调函数中,以处理更复杂的流程。
阅读全文
相关推荐









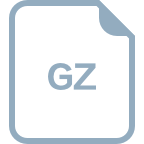

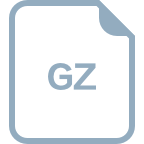





