matlab插值图像怎么写程序
时间: 2024-11-17 15:30:50 浏览: 10
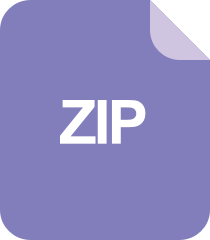
matlab插值讲义和示例程序.zip
在MATLAB中,你可以使用`interpolant`函数来进行图像插值。这里是一个简单的示例,展示如何对一幅图像进行线性插值:
```matlab
% 加载图片
img = imread('your_image_file.jpg'); % 替换为你要处理的实际图片文件名
% 确定插值方法(线性插值)
interp_method = 'linear';
% 创建插值对象
interpolator = imresize(img, [新尺寸1 新尺寸2], interp_method);
% 插值操作
reconstructed_img = resample(interpolator, [原图尺寸1 原图尺寸2]);
% 显示原始和插值后的图像
figure;
subplot(1,2,1), imshow(img);
title('Original Image');
subplot(1,2,2), imshow(reconstructed_img);
title('Interpolated Image');
```
在这个例子中,你需要将`'your_image_file.jpg'`替换为你实际的图像文件路径,同时根据需要调整`新尺寸1`和`新尺寸2`。`imresize`函数用于缩放图像并创建插值对象,而`resample`函数则基于这个对象进行插值。
阅读全文
相关推荐
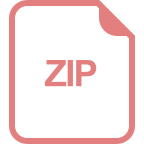
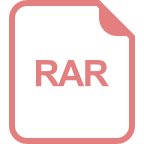
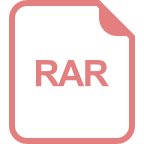
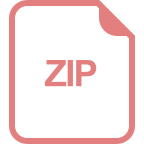
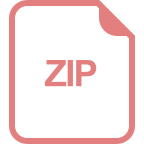
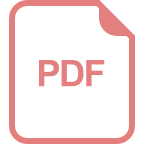
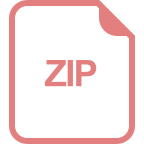
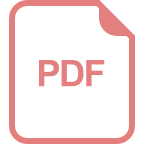
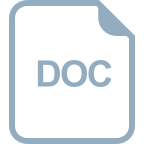
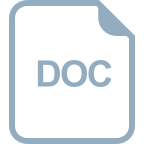
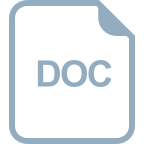
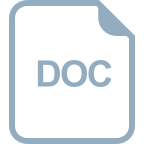
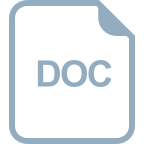
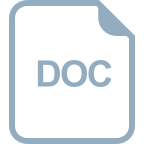

