Python实现图片裁剪
时间: 2023-11-19 16:50:18 浏览: 38
可以使用Python的PIL库(Pillow库)来实现图片裁剪。下面是一个简单的示例代码:
```python
from PIL import Image
def crop_image(image_path, x, y, width, height, output_path):
image = Image.open(image_path)
cropped_image = image.crop((x, y, x+width, y+height))
cropped_image.save(output_path)
# 示例用法
crop_image('input.jpg', 100, 100, 200, 200, 'output.jpg')
```
上述代码中,`crop_image`函数接受五个参数:`image_path`为原始图片的路径,`x`和`y`表示裁剪区域的左上角坐标,`width`和`height`表示裁剪区域的宽度和高度,`output_path`为裁剪后的图片保存路径。
相关问题
python实现图片裁剪代码
以下是使用Python实现图片裁剪的代码示例:
```python
from PIL import Image
def crop_image(image_path, output_path, left, upper, right, lower):
img = Image.open(image_path)
cropped_img = img.crop((left, upper, right, lower))
cropped_img.save(output_path)
# 示例用法
crop_image("./data/cut/thor.jpg", "./data/cut/pil_cut_thor.jpg", 0, 0, 512, 128)
```
这段代码使用了Pillow库来进行图片的裁剪。首先,通过`Image.open()`方法打开原始图片。然后,使用`crop()`方法传入裁剪区域的左上角和右下角坐标来裁剪图片。最后,使用`save()`方法将裁剪后的图片保存到指定路径。在示例中,裁剪区域的左上角坐标为(0, 0),右下角坐标为(512, 128)。
#### 引用[.reference_title]
- *1* [Python实现图片裁剪的两种方式——Pillow和OpenCV](https://blog.csdn.net/hfutdog/article/details/82351549)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [如何用python裁剪图片](https://blog.csdn.net/m0_59485658/article/details/123808484)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Python 实现图片裁剪(附代码) | Python工具](https://blog.csdn.net/zhiweihongyan1/article/details/122605995)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python opencv 图片裁剪
Python通过OpenCV实现图片裁剪是一种常见的图像处理方法。通过引用中的示例代码,可以了解到使用OpenCV库中的cv2.imread()函数加载图像,使用numpy数组进行图像裁剪,使用cv2.imwrite()函数输出裁剪后的图像。同时,引用中还提到了一些注意事项,如参数的位置和类型转换等。如果需要裁剪出不与矩形图片编译平行的图片,可以参考引用中提供的博客进行进一步的改进。
相关推荐
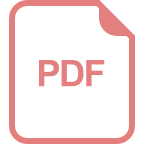
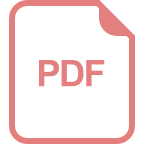












