在C# Winform .NET4.8环境中,如何使用OxyPlot库每秒渲染5000个动态数据点并创建瀑布图?同时,如何实现根据数据点数量自动调整颜色的特性?能否给出一个包括数据生成和颜色映射的详细示例代码?
时间: 2024-10-22 16:13:02 浏览: 27
在C# WinForms .NET4.8环境中使用OxyPlot库来每秒渲染5000个动态数据点并创建瀑布图,同时实现根据数据点数量自动调整颜色的特性,可以通过以下步骤完成:
1. **安装OxyPlot库**:首先需要在你的项目中安装OxyPlot库。你可以通过NuGet包管理器来安装它。
2. **设置WinForms应用程序**:创建一个WinForms应用程序,并在窗体中添加一个`PlotView`控件。
3. **生成数据和更新图表**:编写代码来生成数据并更新图表。
4. **颜色映射**:根据数据点的数量自动调整颜色。
以下是一个完整的示例代码,展示了如何实现上述功能:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using OxyPlot;
using OxyPlot.Series;
using OxyPlot.Axes;
using OxyPlot.WindowsForms;
namespace WaterfallChartExample
{
public partial class Form1 : Form
{
private PlotView plotView;
private LineSeries lineSeries;
private Timer timer;
private Random random = new Random();
private List<double> dataPoints = new List<double>();
private int maxDataPoints = 5000;
public Form1()
{
InitializeComponent();
InitializePlot();
StartDataGeneration();
}
private void InitializePlot()
{
plotView = new PlotView
{
Dock = DockStyle.Fill,
Location = new System.Drawing.Point(0, 0),
Name = "plotView",
Size = new System.Drawing.Size(800, 600),
TabIndex = 0,
Text = "plotView"
};
this.Controls.Add(plotView);
var model = new PlotModel { Title = "Waterfall Chart Example" };
lineSeries = new LineSeries { MarkerType = MarkerType.Circle };
model.Series.Add(lineSeries);
plotView.Model = model;
}
private void StartDataGeneration()
{
timer = new Timer();
timer.Interval = 1000 / 5000; // Generate 5000 points per second
timer.Tick += OnTimerTick;
timer.Start();
}
private void OnTimerTick(object sender, EventArgs e)
{
double newValue = random.NextDouble();
dataPoints.Add(newValue);
if (dataPoints.Count > maxDataPoints)
{
dataPoints.RemoveAt(0);
}
UpdatePlot();
}
private void UpdatePlot()
{
lineSeries.Points.Clear();
for (int i = 0; i < dataPoints.Count; i++)
{
double y = dataPoints[i];
double x = i;
lineSeries.Points.Add(new DataPoint(x, y));
}
plotView.InvalidatePlot(true);
}
}
}
```
### 解释代码:
1. **初始化PlotView和LineSeries**:在`InitializePlot`方法中,我们创建了一个`PlotView`控件并将其添加到窗体中。然后,我们创建了一个`LineSeries`并将其添加到`PlotModel`中。
2. **启动数据生成**:在`StartDataGeneration`方法中,我们设置了一个定时器,每秒钟生成5000个数据点。定时器的间隔设置为`1000 / 5000`毫秒,即每200微秒生成一个数据点。
3. **生成数据并更新图表**:在`OnTimerTick`事件处理程序中,我们生成一个新的随机数据点并将其添加到`dataPoints`列表中。如果数据点的数量超过了最大值(5000),则移除最早的数据点。然后调用`UpdatePlot`方法来更新图表。
4. **更新图表**:在`UpdatePlot`方法中,我们清空了`LineSeries`中的现有数据点,并将新的数据点添加到其中。最后,调用`InvalidatePlot`方法来刷新图表。
阅读全文
相关推荐
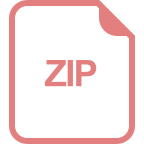
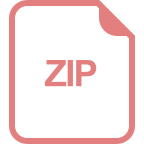
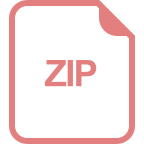
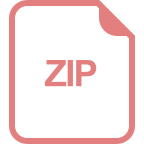
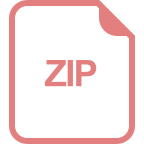
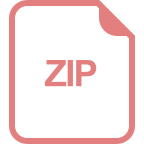
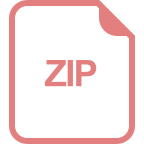
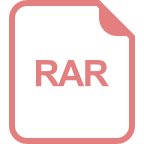
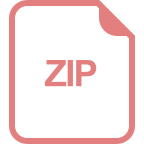
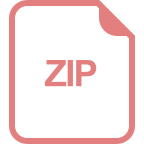
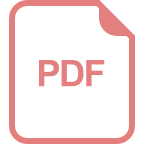
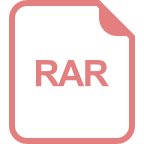
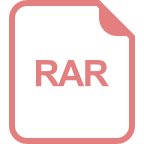
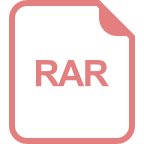
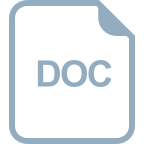
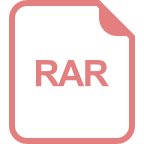
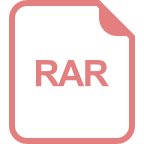
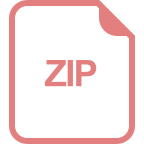
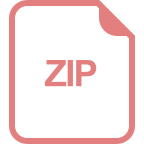