com.itextpdf.text.pdf.PDDocument
时间: 2024-08-13 22:00:23 浏览: 108
`com.itextpdf.text.pdf.PDDocument`是iText库中的一个核心类,它是iText用于处理PDF文档的核心对象。iText是一个开源的Java库,用于生成PDF文档,读取PDF内容,以及对PDF进行各种操作,如添加文本、图像、表单和页眉页脚等。
当你创建一个`PDDocument`实例时,通常意味着你想要开始一个新的PDF文件或打开一个现有的PDF进行操作。`PDDocument`提供了对PDF文件的高级操作接口,例如:
- `PDDocument.open(String fileName)`: 打开一个已存在的PDF文件。
- `PDDocument create(String fileName, boolean append)`: 创建一个新的PDF文件,如果`append`为true,则追加到指定的文件。
- `void close()`: 关闭当前的PDF文档,释放资源。
- `Chapter addChapter(String title)`: 添加一个章节到文档中,用于组织内容。
通过这个类,你可以执行诸如添加新页面、设置字体样式、插入文本和图像等操作,最后记得在完成所有操作后调用`close()`方法来保存并关闭文档。
相关问题
java利用iText 5.5.13或PDFBox2.0.27实现双层PDF转为单层PDF,改为不可搜索的PDF
在Java中,要将双层PDF(包含嵌入字体的文本)转换为单层PDF并使其不可搜索,你可以使用iText库或PDFBox。这里分别提供两个库的简单示例:
### 使用iText 5.5.13
首先确保已经添加了iText依赖。如果你使用Maven,可以在pom.xml中添加:
```xml
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
```
然后,你可以使用以下代码进行转换:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
public class PDFConverter {
public static void main(String[] args) {
try {
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream("output_single_layer.pdf"));
// 设置页面属性为不可搜索
PdfAction action = new PdfAction();
action.setSuspendIsometric(true);
PdfStamper stamper = new PdfStamper(document, new FileOutputStream("output_searchable.pdf"), true);
PdfPage page = stamper.getOverContent(1); // 获取第一页
page.addAnnotation(PdfAnnotation.HIGHLIGHTING, 0, 0, action);
// 将文档写入单层PDF
document.open();
document.close();
// 另外一个文件作为模板,复制到单层PDF并替换嵌入字体
PdfReader reader = new PdfReader("template_with_fonts.pdf");
PdfCopy copy = new PdfCopy(stamper, reader);
copy.copyPageRange(1, reader.getNumberOfPages());
reader.close();
stamper.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
### 使用PDFBox 2.0.27
PDFBox也有相似的功能,你需要先添加依赖:
```xml
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.27</version>
</dependency>
```
代码示例如下:
```java
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
public class PDFBoxConverter {
public static void main(String[] args) {
try {
PDDocument originalDocument = PDDocument.load(new File("input_double_layer.pdf"));
// 创建一个新的PDDocument,用于存储结果
PDDocument outputDocument = new PDDocument();
PDFTextStripper stripper = new PDFTextStripper();
stripper.setEmbedAllFonts(false); // 关闭字体嵌入
// 遍历每一页,并提取文本
for (int i = 1; i <= originalDocument.getNumberOfPages(); i++) {
PDPage page = originalDocument.getPage(i);
PDAcroForm acroForm = page.getAcroForm();
if (acroForm != null) { // 检查是否存在表单,如果有则清除
acroForm.clear();
}
PDFTextStripper.TextToImageWorker worker = new PDFTextStripper.TextToImageWorker(page);
BufferedImage image = worker.getImage();
ImageIO.write(image, "png", new File("temp_page_" + i + ".png")); // 写入临时图片
// 将文本直接写入新文档,避免保留原始字体
PDFTextStripper strip = new PDFTextStripper();
String text = strip.getText(page);
outputDocument.addPage(PDFTextUtil.createPDFTextPage(text));
// 清理资源
worker.dispose();
image.flush();
image.close();
}
// 将输出文档保存为不可搜索的PDF
outputDocument.save("output_single_layer.pdf");
outputDocument.close();
originalDocument.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
pdfbox&iText生成PDF文件格式及读取PDF文件内容的小示例--完美支持中文版
好的,我可以为您提供一个关于使用pdfbox和iText生成PDF文件格式及读取PDF文件内容的小示例,完美支持中文版。
首先,您需要安装pdfbox和iText相关的依赖库。您可以在Maven中央仓库中找到这些依赖项,或者直接从官方网站下载相应的jar包。接下来,您可以按照如下步骤进行操作:
生成PDF文件格式:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
import org.apache.pdfbox.pdmodel.edit.PDPageContentStream;
public class PDFGenerator {
public static void main(String[] args) {
try {
PDDocument doc = new PDDocument();
PDPage page = new PDPage();
doc.addPage(page);
PDPageContentStream content = new PDPageContentStream(doc, page);
content.beginText();
content.setFont(PDType1Font.HELVETICA_BOLD, 12);
content.moveTextPositionByAmount(100, 700);
content.drawString("Hello World!");
content.endText();
content.close();
doc.save(new File("HelloWorld.pdf"));
doc.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们创建了一个PDDocument对象,然后添加了一个PDPage对象。接下来,我们创建了一个PDPageContentStream对象,将字体设置为Helvetica Bold,将文本位置移动到(100, 700),然后绘制了一个字符串“Hello World!”。最后,我们关闭了PDPageContentStream对象,并将PDDocument保存到一个名为“HelloWorld.pdf”的文件中。
读取PDF文件内容:
```java
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
public class PDFReader {
public static void main(String[] args) {
try {
PDDocument doc = PDDocument.load(new File("HelloWorld.pdf"));
PDFTextStripper stripper = new PDFTextStripper();
String text = stripper.getText(doc);
System.out.println(text);
doc.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们加载了之前生成的PDF文件,并使用PDFTextStripper对象提取了文本内容。最后,我们将提取的文本内容打印到控制台上。
希望这个小示例能够帮助到您!
阅读全文
相关推荐
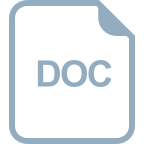
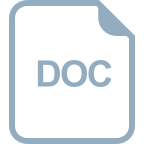
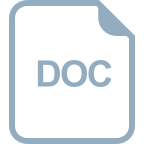
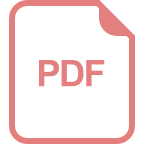
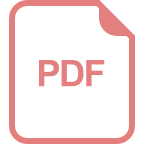
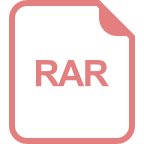
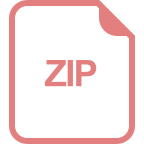
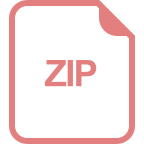
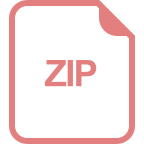
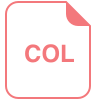
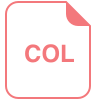





