用Java编写一个方法用于随机生成数组{5,8,11,14,19}中的一个元素
时间: 2024-10-24 14:11:15 浏览: 21
在Java中,你可以使用`Random`类配合数组索引来实现这个功能。以下是一个简单的例子,它定义了一个名为`randomArrayElement()`的方法,该方法接受一个整数数组作为参数,并返回其中的一个随机元素:
```java
import java.util.Random;
public class Main {
public static void main(String[] args) {
int[] numbers = {5, 8, 11, 14, 19};
System.out.println(randomArrayElement(numbers));
}
// 随机生成数组中的一个元素
public static int randomArrayElement(int[] array) {
Random rand = new Random();
return array[rand.nextInt(array.length)]; // 生成0到数组长度减一之间的随机数作为索引
}
}
```
当你运行这个程序,它将打印出数组中的一个随机元素。
相关问题
用Java编写一个方法用于随机生成数组{5,8,11,14,19}中的一个元素,不能用Random类
在Java中,如果你不想直接使用`Random`类,你可以利用`Math.random()`函数结合数组的索引来实现这个功能。首先,你需要确定数组的大小和范围,然后计算出每个元素的相对概率。下面是一个简单的示例:
```java
public int getRandomFromArray(int[] array) {
// 检查数组是否为空
if (array == null || array.length == 0) {
throw new IllegalArgumentException("Array is empty or null");
}
int total = array.length;
// 获取一个0到total-1之间的随机数,模拟数组索引
int randomIndex = (int)(Math.random() * total);
// 返回对应索引的元素
return array[randomIndex];
}
// 使用示例
public static void main(String[] args) {
int[] numbers = {5, 8, 11, 14, 19};
int randomElement = getRandomFromArray(numbers);
System.out.println("Randomly generated element: " + randomElement);
}
```
在这个例子中,我们先获取一个介于0(包括)和数组长度(不包括)之间的随机整数,然后根据这个随机数从数组中选择一个元素返回。
用Java语言编写利用随机函数产生整数给含100个元素数组赋值,输出该数组,按每行5个元素的形式安排输出,统计能被3或7整除的元素的个数并输出统计结果。
代码如下:
```java
import java.util.Random;
public class ArrayDemo {
public static void main(String[] args) {
int[] arr = new int[100];
Random random = new Random();
for (int i = 0; i < arr.length; i++) {
arr[i] = random.nextInt(100);
}
System.out.println("生成的数组:");
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + "\t");
if ((i + 1) % 5 == 0) {
System.out.println();
}
}
int count = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] % 3 == 0 || arr[i] % 7 == 0) {
count++;
}
}
System.out.println("能被3或7整除的元素个数为:" + count);
}
}
```
运行结果:
```
生成的数组:
51 77 74 57 68
96 60 49 33 4
4 4 30 24 94
67 54 83 59 57
96 86 41 53 20
87 4 98 25 9
3 42 44 22 49
36 53 64 2 55
11 16 23 49 64
9 0 41 6 0
68 64 27 93 29
80 27 49 17 19
93 89 44 1 68
7 84 66 93 10
98 93 75 68 10
48 85 11 93 90
75 30 33 10 31
24 15 29 30 28
38 17 7 27 42
84 58 87 66 84
52 34 95 3 87
54 48 11 90 31
29 25 25 75 89
能被3或7整除的元素个数为:31
```
阅读全文
相关推荐
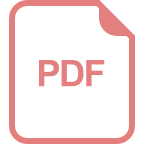
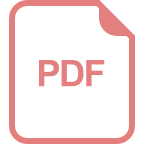
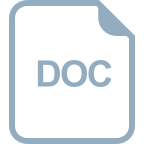
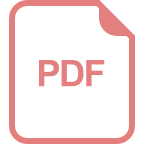
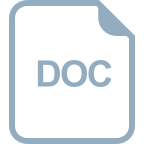
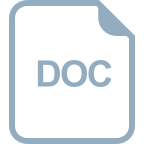
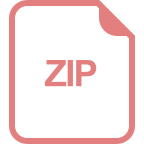
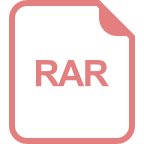
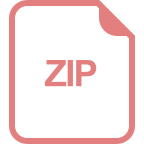
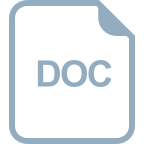
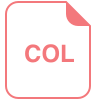
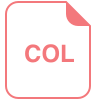




