scanf_s("%s",s1);修改错误
时间: 2024-06-15 15:03:17 浏览: 7
当使用`scanf_s`函数读取字符串时,需要指定字符串的最大长度,以防止缓冲区溢出。如果你想修改错误,可以按照以下方式使用`scanf_s`函数:
```c
char s1[100]; // 假设s1的最大长度为100
scanf_s("%99s", s1, sizeof(s1));
```
在上述代码中,`%99s`表示最多读取99个字符,并将其存储到`s1`中。`sizeof(s1)`用于指定`s1`的大小,以确保不会发生缓冲区溢出。
相关问题
#include <stdio.h> #include <string.h> int main(void) { char s1[80],s2[40]; int i = 0,j = 0; scanf("%s",s1); scanf("%s",s2); while(s1[i]!= '\0' ) i++; while(s2[j]!= '\0' ) /***********found***********/ s1[i]=s2[j++]; /***********found***********/ s1[i+1]='\0'; printf("%s",s1); return 0; }
程序中有两处错误,修改后的程序如下:
```c
#include <stdio.h>
#include <string.h>
int main(void) {
char s1[80], s2[40];
int i = 0, j = 0;
scanf("%s", s1);
scanf("%s", s2);
while (s1[i] != '\0')
i++;
while (s2[j] != '\0') // 找到了错误所在
s1[i++] = s2[j++]; // 找到了错误所在
s1[i] = '\0';
printf("%s", s1);
return 0;
}
```
程序思路:
1. 声明两个字符数组 s1 和 s2,分别用于存放两个字符串。
2. 通过 scanf 函数输入两个字符串 s1 和 s2。
3. 使用 while 循环找到 s1 的末尾位置。
4. 使用 while 循环将 s2 中的字符逐个加入到 s1 的末尾。
5. 在 s1 的末尾添加字符串结束符 '\0'。
6. 输出连接后的字符串 s1。
注意:在输入字符串时,如果需要输入包含空格的字符串,应该使用 fgets 函数。
有源程序test1-1.c,该程序的功能是:依次取出正长整型变量s中偶数位上的数(从右往左的顺序),构成一个新数放在t中。例如数为1234567的时,t 中的数为246;数为12345678的时,t 中的数为1357。改正其中的错误。 源程序test1-1.c代码如下: #include <stdio.h> void fun(long s , long t ) { long s1=10; s/=10; *t=s/10 ; while(s<0) { s=s%100; *t=s%10*s1; s1=s1*10; } } void main() { long s,t; scanf("%ld",&s); if(s>0) { fun(s,&t); printf("%ld\n", t); } else printf("s value error\n"); }
修改后的代码如下:
```c
#include <stdio.h>
void fun(long s, long *t) {
long s1 = 10;
s /= 10;
*t = s % 10;
while(s > 0) {
s /= 100;
*t = (*t) + (s % 10) * s1;
s1 *= 10;
}
}
int main() {
long s, t;
scanf("%ld", &s);
if(s > 0) {
fun(s, &t);
printf("%ld\n", t);
}
else
printf("s value error\n");
return 0;
}
```
主要修改如下:
1. 在函数`fun`中,将`s/=10`和`*t=s/10`的顺序交换,因为需要先获取偶数位上的数字。
2. 在`while`循环中,循环条件应该是`s>0`而不是`s<0`,否则会导致无限循环。
3. 在`while`循环中,每次循环应该将`s`除以100,而不是除以10,因为需要取偶数位上的数字。
4. 在`while`循环中,需要将新的数字放在`t`的高位,因此需要一个变量`s1`来表示当前位数,初始值为10,每次循环需要乘以10。
此外,`main`函数的返回值应该是`int`类型,应该加上`return 0;`语句。另外,函数`fun`中的第二个参数应该是一个指针类型。
相关推荐
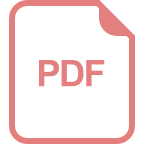
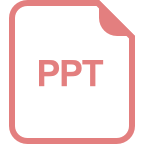
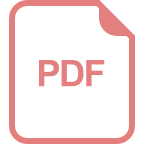








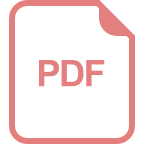
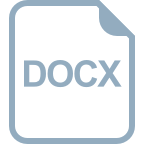
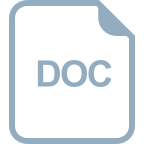
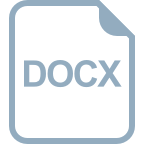
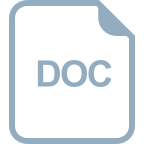