怎么在嵌套list和dict中找到特定的值
时间: 2024-10-16 21:21:14 浏览: 17
在Python中,查找嵌套列表和字典中的特定值涉及到递归搜索。以下是几种常见的方法:
1. **使用循环和条件判断**:
对于嵌套列表,你可以遍历每个元素,如果是列表则继续递归,如果是字典,则检查其键是否匹配目标值。
```python
def find_value(nested_list, value):
for item in nested_list:
if isinstance(item, list):
if find_value(item, value): return True
elif isinstance(item, dict) and item.get('key') == value:
return True
return False
```
2. **使用递归函数**:
可以定义一个专门的递归函数来处理这个问题,如果遇到字典,检查值;如果遇到列表,再对其中的元素递归查找。
```python
def search_nested(nested, target, path=[]):
if isinstance(nested, dict):
for k, v in nested.items():
new_path = path + [k]
if v == target or search_nested(v, target, new_path):
return True
elif isinstance(nested, list):
for i, val in enumerate(nested):
if val == target or search_nested(val, target, path + [i]):
return True
return False
```
3. **使用内置函数**:
使用`any()`和`lambda`表达式结合递归也是可行的,这样更简洁一些。
```python
def find_in_nested(data, value):
return any(value == v for d in data.values() for v in (d.values() if isinstance(d, dict) else d))
```
要查找特定值,只需调用上述函数并传入你的嵌套数据和目标值:
```python
nested_data = {'a': [1, 2, {'b': 'target'}], 'c': {'d': 'another'}}
value_to_find = 'target'
result = search_nested(nested_data, value_to_find)
```
阅读全文
相关推荐
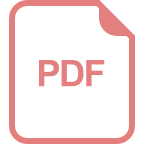
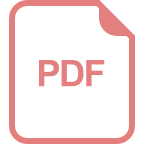
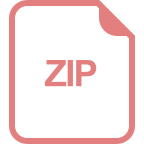
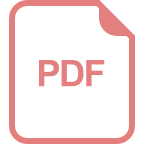
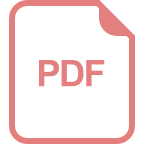
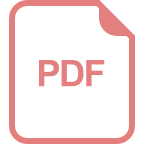
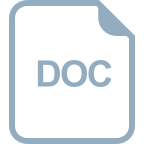
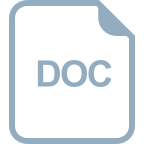
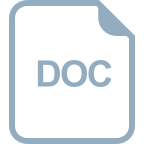
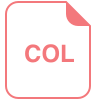
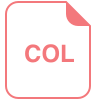
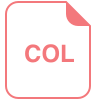
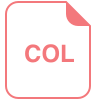
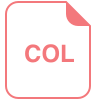
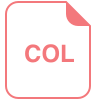
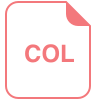
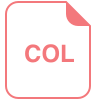
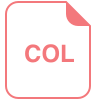
