AES 加密解密 java
时间: 2024-01-29 14:10:15 浏览: 41
以下是使用AES算法实现加密和解密的Java代码示例:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class AESUtils {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
public static String encrypt(String plaintext, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String ciphertext, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(ciphertext));
return new String(decryptedBytes, StandardCharsets.UTF_8);
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
try {
String plaintext = "Hello, World!";
String key = "0123456789abcdef"; // 16字节的密钥
String ciphertext = AESUtils.encrypt(plaintext, key);
System.out.println("Encrypted: " + ciphertext);
String decryptedText = AESUtils.decrypt(ciphertext, key);
System.out.println("Decrypted: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
注意:在实际使用中,请确保密钥的安全性和合法性,并根据具体需求选择合适的加密模式和填充方式。
相关推荐
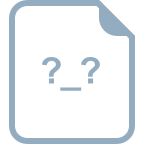














