C++ 函数的应用举例
时间: 2023-11-08 11:04:07 浏览: 143
当然!C++ 函数可以应用于各种不同的场景和问题。下面是一些常见的 C++ 函数的应用举例:
1. 数学计算:C++ 函数可以用于执行各种数学计算,如求平方根、取绝对值、计算三角函数等。
```cpp
#include <iostream>
#include <cmath>
int main() {
double x = 4.0;
double squareRoot = sqrt(x);
double absoluteValue = abs(-10);
double sineValue = sin(30 * M_PI / 180); // 将角度转换为弧度
std::cout << "Square root: " << squareRoot << std::endl;
std::cout << "Absolute value: " << absoluteValue << std::endl;
std::cout << "Sine value: " << sineValue << std::endl;
return 0;
}
```
2. 字符串处理:C++ 函数可以用于对字符串进行各种操作,例如查找子串、替换字符、转换大小写等。
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
int index = str.find("world"); // 查找子串的位置
str.replace(index, 5, "C++"); // 替换子串
for (char& c : str) {
c = toupper(c); // 转换为大写
}
std::cout << str << std::endl;
return 0;
}
```
3. 文件操作:C++ 函数可以用于读取和写入文件,以及对文件进行其他操作,如复制、移动、删除等。
```cpp
#include <iostream>
#include <fstream>
int main() {
std::ifstream inputFile("input.txt"); // 打开输入文件
std::ofstream outputFile("output.txt"); // 打开输出文件
if (inputFile && outputFile) {
std::string line;
while (std::getline(inputFile, line)) {
outputFile << line << std::endl; // 将每行写入输出文件
}
inputFile.close(); // 关闭输入文件
outputFile.close(); // 关闭输出文件
} else {
std::cout << "Failed to open files." << std::endl;
}
return 0;
}
```
这些只是 C++ 函数的一小部分应用举例,函数的应用范围非常广泛,可以根据具体需求进行灵活运用。
阅读全文
相关推荐
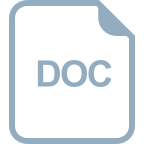
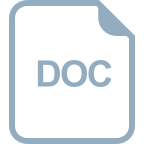
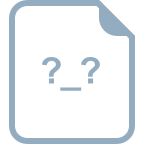
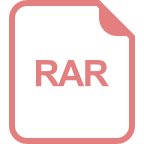
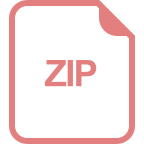
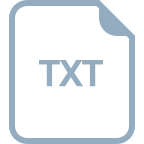
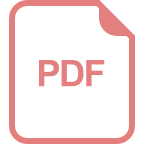
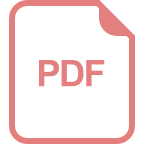
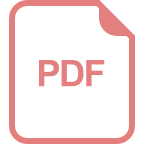
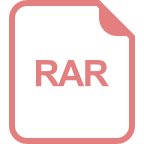
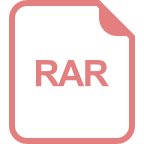
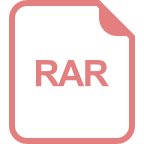
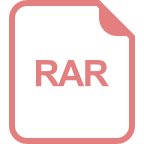
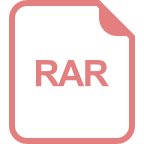
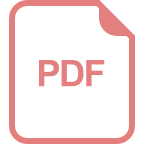