Java完成链栈的求长度和display操作
时间: 2024-10-20 10:05:07 浏览: 21
在Java中,我们可以使用链表数据结构来实现链栈(Linked Stack),其中节点包含了值和指向下一个节点的引用。对于链栈的长度求解(即元素个数)和显示(即打印栈的内容),可以这样做:
**链栈节点类(Node)**:
```java
public class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
```
**链栈类(LinkedStack)**:
```java
public class LinkedStack {
private Node top;
// 添加元素到栈顶
public void push(int value) {
Node newNode = new Node(value);
newNode.next = top;
top = newNode;
}
// 删除并返回栈顶元素(长度+1)
public int pop() {
if (top == null) {
throw new IllegalStateException("Stack is empty");
}
int poppedValue = top.data;
top = top.next;
return poppedValue;
}
// 返回栈顶元素但不删除(长度不变)
public int peek() {
if (top == null) {
throw new IllegalStateException("Stack is empty");
}
return top.data;
}
// 求栈的长度(元素个数)
public int size() {
int count = 0;
Node current = top;
while (current != null) {
count++;
current = current.next;
}
return count;
}
// 显示链栈内容
public void display() {
if (top == null) {
System.out.println("Stack is empty");
return;
}
Node temp = top;
while (temp != null) {
System.out.print(temp.data + " ");
temp = temp.next;
}
System.out.println();
}
}
```
阅读全文
相关推荐
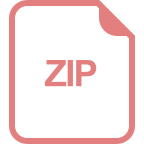
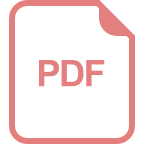
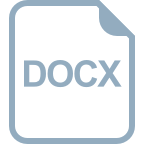
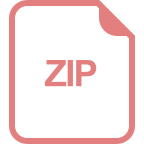
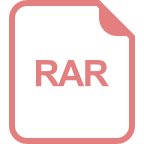
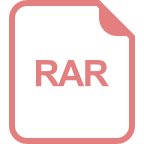
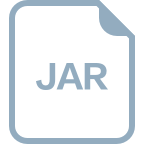
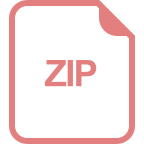
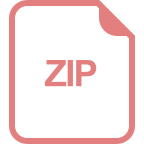
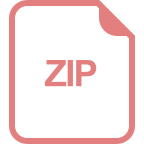
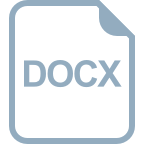
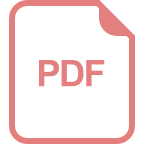
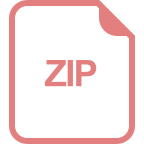
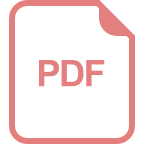
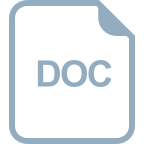
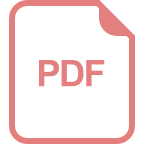
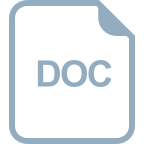
