编写main.c模块,在main函数中调用上面的copy函数,将一个文件拷贝为另一个文件。
时间: 2024-11-09 11:18:30 浏览: 5
在`main.c`模块中,你可以直接包含`my_copy.c`文件,并在`main`函数中调用`copy_file`函数。假设你已经包含了必要的路径信息,下面是如何做到这一点的一个基本示例:
```c
#include "my_copy.c" // 如果文件在同一目录下,或者有正确的包含路径
int main() {
const char* src_file = "path_to_original_file"; // 替换为你源文件的实际路径
const char* dest_file = "path_to_destination_file"; // 替换为你目标文件的实际路径
if (copy_file(src_file, dest_file) != 0) { // 调用copy_file并检查返回值
printf("Failed to copy the file.\n");
return 1; // 返回错误码
} else {
printf("File copied successfully.\n");
return 0; // 成功复制,返回0
}
}
相关问题
编写一个cuicopy.py文件,调用hit.py模块的copy函数,实现命令行文件拷贝功能并实时输出百分比进度。cuicopy.py运行时以命令行参数的形式传入源文件和目标文件路径,运行如下图:
要在Python中编写`cuicopy.py`文件,实现命令行下的文件拷贝功能,并显示进度百分比,你可以使用`shutil`库提供的`copyfileobj()`函数配合`tqdm`库来展示进度条。首先确保已经安装了`tqdm`库,如果没有安装,可以使用`pip install tqdm`命令。
下面是一个简单的`cuicopy.py`文件示例:
```python
import shutil
from tqdm import tqdm
def copy_with_progress(src_path, dest_path):
with open(src_path, 'rb') as src_file, open(dest_path, 'wb') as dest_file:
byte_amount = os.path.getsize(src_path)
progress_bar = tqdm(total=byte_amount, unit='B', unit_scale=True, desc="Copying")
while True:
data = src_file.read(4096)
if not data:
break
dest_file.write(data)
progress_bar.update(len(data))
if __name__ == "__main__":
import argparse
parser = argparse.ArgumentParser(description='Copy a file with progress bar.')
parser.add_argument('src', help='Source file path')
parser.add_argument('dest', help='Destination file path')
args = parser.parse_args()
try:
copy_with_progress(args.src, args.dest)
print(f"File copied successfully to {args.dest}")
except Exception as e:
print(f"Error occurred: {e}")
```
运行`cuicopy.py`时,在命令行输入类似这样的格式:
```bash
python cuicopy.py /path/to/source/file source.txt /path/to/destination/file destination.txt
```
这将会拷贝源文件到目标文件,并显示进度条。
编写一函数,将一个字符串拷贝至另一个字符串中,要求用字符指针实现(不能使用strcpy函数)。在主函数中输入任意字符串,调用该函数之后输出拷贝后的字符串。C语言
下面是实现该功能的代码:
```c
#include <stdio.h>
void copy_string(char *from, char *to)
{
while (*from != '\0')
{
*to = *from;
++from;
++to;
}
*to = '\0'; // 添加字符串结尾标志
}
int main()
{
char str1[100], str2[100];
printf("请输入一个字符串:");
scanf("%s", str1);
copy_string(str1, str2);
printf("拷贝后的字符串为:%s\n", str2);
return 0;
}
```
首先定义了一个 `copy_string` 函数,该函数接受两个字符指针参数,分别指向源字符串和目标字符串。该函数通过循环遍历源字符串中的每个字符,并将其逐个拷贝到目标字符串中。最后添加一个字符串结尾标志 `\0`。
在主函数中,首先输入一个字符串,然后调用 `copy_string` 函数将其拷贝到另外一个字符串中。最后输出拷贝后的字符串即可。
阅读全文
相关推荐
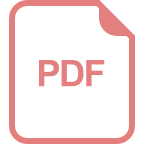
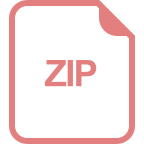
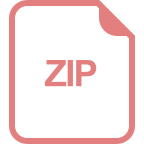










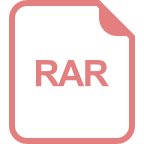