编写一个能计算表达式值的小程序。 相关知识 为了完成本关任务,你需要掌握:1.栈的封装,2.字符串的遍历,3,表达式求值的原理。 C语言代码全部
时间: 2024-09-29 22:11:40 浏览: 44
编写一个能计算表达式值的小程序通常涉及到解析和计算逆波兰表示法(Reverse Polish Notation,RPN),因为这种方式可以避免括号带来的复杂性。这里是一个简单的C语言版本,它利用栈来实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
double num;
} StackElement;
#define MAX_STACK_SIZE 100
StackElement stack[MAX_STACK_SIZE];
int top = -1;
double get_num(char *token) {
long val = strtol(token, NULL, 10);
return (val == LONG_MIN || val == LONG_MAX) ? 0 : (double)val;
}
void push(double val) {
if (top < MAX_STACK_SIZE - 1) {
stack[++top] = (StackElement){val};
} else {
printf("Error: Stack overflow\n");
}
}
double pop() {
if (top >= 0) {
return stack[top--].num;
} else {
printf("Error: Stack underflow\n");
return 0;
}
}
double evaluate_expression(char* expression) {
char token[100], current;
int i = 0;
while (expression[i]) {
if (isdigit(expression[i]) || expression[i] == '.') {
strcpy(token, &expression[i]);
while (isdigit(expression[i + 1])) {
i++;
}
i++; // Skip the digit after the number
push(get_num(token));
} else if (expression[i] == '+' || expression[i] == '-') {
double op2 = pop();
double op1 = pop();
if (expression[i] == '+') {
push(op1 + op2);
} else {
push(op1 - op2);
}
} else if (expression[i] == '*' || expression[i] == '/') {
double op2 = pop();
double op1 = pop();
if (expression[i] == '*') {
push(op1 * op2);
} else {
push(op1 / op2);
}
} else if (expression[i] == '(') {
i++; // Skip opening parenthesis
} else if (expression[i] == ')') {
break; // End of expression when closing parenthesis found
} else {
printf("Invalid character at index %d\n", i);
}
i++;
}
return pop(); // Return the final result
}
int main() {
char expr[] = "3.14 * 5 + 7 / 2"; // Example input
double result = evaluate_expression(expr);
printf("The value of the expression is %.2f\n", result);
return 0;
}
```
这个程序首先将输入的字符串按字符分割,处理数字、运算符和括号,然后利用栈的数据结构存储数值并执行相应的加减乘除操作。注意这只是一个基础示例,实际项目中可能会需要更复杂的语法分析。
阅读全文
相关推荐
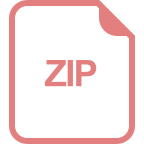
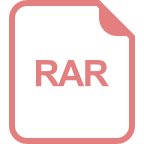
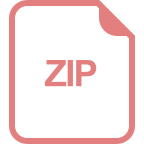


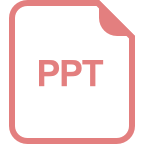
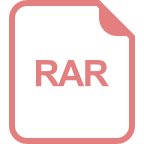
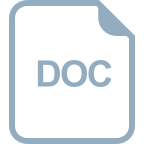
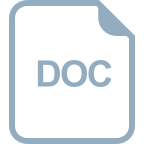
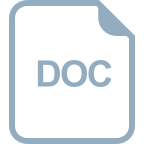
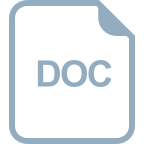
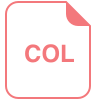
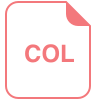
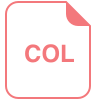
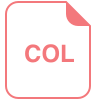
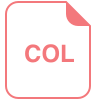
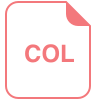
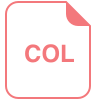
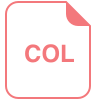