栈在编程中的应用:表达式求值与算法优化
发布时间: 2024-09-12 18:41:47 阅读量: 109 订阅数: 26 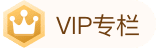
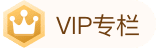
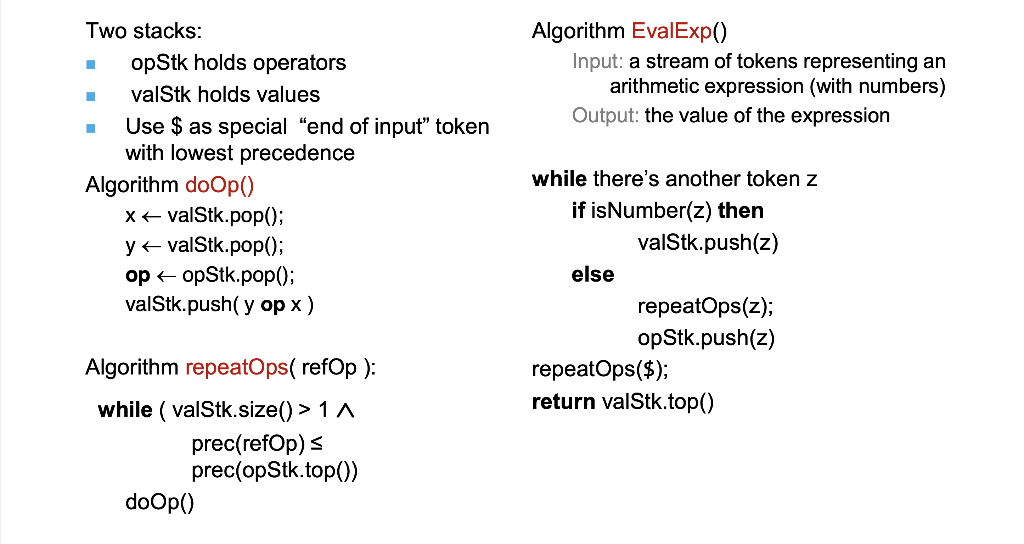
# 1. 栈的基本概念和原理
## 简介
栈是一种特殊的线性数据结构,遵循后进先出(LIFO)的原则。本章节将介绍栈的基本概念、性质和基本操作,为理解后续章节中的高级应用和算法实现打下坚实的基础。
## 栈的定义与性质
栈可以视为一种“只能在一端添加或删除元素”的数组或链表。它主要由两个操作构成:`push`(进栈)和`pop`(出栈)。其中,`push`操作将元素添加到栈顶,`pop`操作则移除栈顶元素。
## 栈的操作与应用场景
- `peek`:查看栈顶元素而不移除它。
- `isEmpty`:检查栈是否为空。
栈的应用场景非常广泛,从函数调用栈、递归算法的实现,到支持后退历史的浏览器等都涉及到栈的使用。
# 2. 表达式求值的栈实现
在探讨栈的实际应用时,表达式求值是一个将理论知识转化为实践操作的经典案例。本章节将逐步深入表达式求值中栈的应用,解释理论基础,并提供应用示例。
## 2.1 表达式求值的理论基础
在着手编写代码或使用栈进行表达式求值之前,有必要了解一些基础理论知识。
### 2.1.1 逆波兰表示法的介绍
逆波兰表示法(Reverse Polish Notation,RPN),也称为后缀表示法,是一种去掉括号的数学表达式写法。在RPN中,运算符位于与之相关的操作数之后,这使得计算过程可以顺序进行,无需括号。
例如,中缀表达式 `(3 + 4) * 5` 可以被转换为后缀表达式 `3 4 + 5 *`。
使用栈来实现后缀表达式的计算,是因为后缀表达式具有从左到右的线性顺序,符合栈的后进先出(LIFO)特性。
### 2.1.2 中缀表达式向后缀表达式的转换
将中缀表达式转换为后缀表达式通常需要使用两个栈:一个用于操作符(运算符栈),另一个用于输出(输出栈)。转换算法的步骤如下:
1. 初始化两个栈:运算符栈和输出栈。
2. 从左到右扫描中缀表达式。
3. 遇到操作数时,直接将其压入输出栈。
4. 遇到运算符时,根据运算符的优先级和栈顶运算符的优先级,决定是否进行出栈和入栈操作。
5. 遇到左括号时,直接将运算符压入运算符栈。
6. 遇到右括号时,依次弹出运算符栈顶运算符,并输出到输出栈,直到遇到左括号为止,然后弹出左括号。
7. 表达式扫描完毕后,依次弹出运算符栈中剩余的运算符,并输出。
下面是一个将中缀表达式转换为后缀表达式的伪代码示例:
```pseudo
function infixToPostfix(infixExpr):
operatorStack = new Stack() // 初始化运算符栈
postfixList = [] // 初始化输出列表
for each token in infixExpr:
if token is operand:
postfixList.append(token)
else if token is '(':
operatorStack.push(token)
else if token is ')':
*** is not '(':
postfixList.append(operatorStack.pop())
operatorStack.pop() // 弹出 '('
else if token is operator:
while not operatorStack.isEmpty() and hasPrecedence(token, ***()):
postfixList.append(operatorStack.pop())
operatorStack.push(token)
while not operatorStack.isEmpty():
postfixList.append(operatorStack.pop())
return postfixList
function hasPrecedence(op1, op2):
return (op1 is '(' and op2 is not '(') or
(precedence of op1 is greater than or equal to precedence of op2)
```
## 2.2 栈在表达式求值中的应用
接下来,将探讨如何利用栈来完成实际的表达式求值。
### 2.2.1 栈用于处理括号匹配
在表达式求值时,括号匹配是必须首先解决的问题。通过栈,我们可以检查每对括号是否正确匹配。以下是一个简单的括号匹配算法的伪代码:
```pseudo
function isMatchingPair(expr):
stack = new Stack()
for each character in expr:
if character is '(':
stack.push(character)
else if character is ')':
if stack.isEmpty():
return False
else:
stack.pop()
return stack.isEmpty()
```
### 2.2.2 栈用于计算后缀表达式
一旦我们有了后缀表达式,就可以用栈来计算它的值。计算后缀表达式的步骤如下:
1. 初始化一个空栈。
2. 从左到右扫描后缀表达式。
3. 遇到操作数时,将其压入栈中。
4. 遇到运算符时,从栈中弹出所需数量的操作数,进行计算,再将结果压入栈中。
5. 表达式扫描完毕后,栈顶元素即为最终结果。
伪代码实现如下:
```pseudo
function evaluatePostfix(expression):
stack = new Stack()
for each token in expression:
if token is operand:
stack.push(token)
else if token is operator:
operand2 = stack.pop()
operand1 = stack.pop()
result = performOperation(operand1, operand2, token)
stack.push(result)
return stack.pop()
function performOperation(operand1, operand2, operator):
switch operator:
case '+': return operand1 + operand2
case '-': return operand1 - operand2
case '*': return operand1 * operand2
case '/': return operand1 / operand2
```
### 2.2.3 复杂表达式求值的案例分析
为了加深理解,我们以一个复杂的表达式求值为例。考虑中缀表达式 `((a+b)*(c^d-e))^(f+g*h)-i`。下面展示将此中缀表达式转换为后缀表达式,然后计算其值的过程。
1. 中缀到后缀的转换:
- 扫描到 `(`,将 `(` 压入栈。
- 遇到 `a`,直接输出。
- 遇到 `+`,将 `+` 压入栈。
- 扫描到 `(`,将 `(` 压入栈。
- 遇到 `b`,直接输出。
- 遇到 `)`,弹出栈顶 `)`,输出。
- 遇到 `*`,弹出 `(` 和 `+`,输出,再将 `*` 压入栈。
- 遇到 `c`,直接输出。
- 遇到 `^`,将 `^` 压入栈。
- 扫描到 `d`,直接输出。
- 遇到 `-`,弹出栈顶 `^`,输出,再将 `-` 压入栈。
- 遇到 `e`,直接输出。
- 遇到 `)`,弹出栈顶 `)`,输出。
- 遇到 `^`,弹出栈顶 `*` 和 `-`,输出,再将 `^` 压入栈。
- 扫描到 `(`,将 `(` 压入栈。
- 遇到 `f`,直接输出。
- 遇到 `+`,将 `+` 压入栈。
- 扫描到 `g`,直接输出。
- 遇到 `*`,将 `*` 压入栈。
- 扫描到 `h`,直接输出。
- 遇到 `)`,弹出栈顶 `)`,输出。
- 遇到 `-`,弹出栈顶 `+` 和 `^`,输出,再将 `-` 压入栈。
- 遇到 `i`,直接输出。
- 表达式扫描完毕,弹出栈顶 `-` 和 `^`,输出。
最终得到的后缀表达式为 `a b + c d ^ e - * f g h * + ^ - i -`
2. 计算后缀表达式的值:
这里需要运用上述计算后缀表达式的算法,最终得到具体的数值结果。实际操作中,可以用上述 `evaluatePostfix` 函数,传入计算的后缀表达式。
通过以上的步骤,我们不仅能够理解表达式求值的栈实现原理,而且学会了如何将中缀表达式转换为后缀表达式,并计算后缀表达式的值。栈的使用,使原本复杂且易错的表达式求值过程变得简单和条理清晰。
# 3. 算法优化中的栈应用
在计算机科学中,栈不仅仅是一个数据结构,它在算法设计和优化中扮演着关键角色。特别是在处理递归算法和需要后进先出(LIFO)策略的场景中,栈提供了优雅的解决方案。本章节探讨了栈在排序算法和算法问题解决中的应用,以及如何利用栈实现更高效、更简洁的算法逻辑。
## 3.1 栈在排序算法中的优化
### 3.1.1 堆栈排序的概念和原理
堆栈排序(Stack Sort)是一种基于栈操作实现的排序算法。它的基本思想是使用两个栈,一个栈用于输入元素,另一个用于输出。当输入栈中有元素时,将它推入输出栈,但是只有当输出栈的栈顶元素小于该元素时才执行这一操作。这样,当元素最终被推回输入栈时,它会位于所有较小的元素之上,从而达到排序的目的。
堆栈排序的优势在于它能够在O(n)的空间复杂度下完成排序,而且实现起来非常直观。然而,它的平均和最坏情况时间复杂度为O(n^2),所以它并不是一个高效的排序算法,但作为一种理论上的探索,它加深了我们对排序算法和栈操作的理解。
### 3.1.2 栈排序算法的实现
下面展示了使用Python实现的栈排序算法的一个基本版本:
```python
def stack_sort(arr):
input_stack = arr[:]
output_stack = []
while input_stack:
item = input_stack.pop()
while output_stack and item < output_stack[-1]:
input_stack.append(output_stack.pop())
output_stack.append(item)
return output_stack
# 示例数组
array = [5, 2, 9, 1, 5, 6]
sort
```
0
0
相关推荐
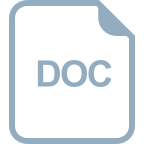
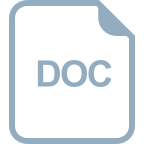
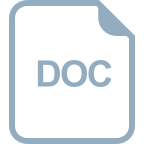
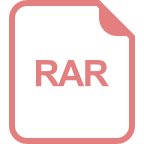
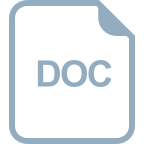
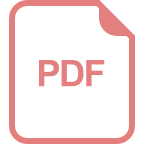
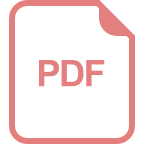
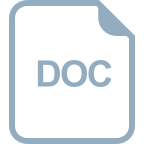
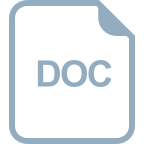