递归数据结构揭秘:二叉树遍历的深入讲解
发布时间: 2024-09-12 19:02:03 阅读量: 41 订阅数: 36 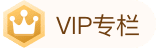
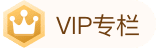
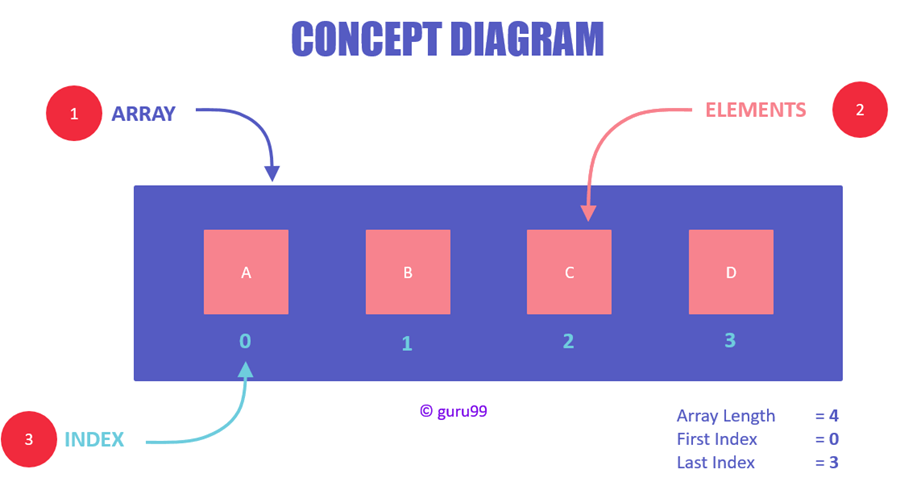
# 1. 二叉树的基本概念与性质
## 1.1 二叉树的定义
二叉树是数据结构领域中一种特别重要的非线性数据结构,具有树形结构的特征。每个节点最多有两个子节点,分别是左子节点和右子节点。若一个二叉树的每个节点都恰好有0个或者2个子节点,则称这种二叉树为满二叉树。如果一个二叉树除最后一层外,其余层的节点数都是满的,且最后一层的节点都靠左排列,这样的二叉树称为完全二叉树。
## 1.2 二叉树的性质
二叉树拥有许多有趣的性质,这些性质在算法优化和问题解决中非常有用。例如,一个有n个节点的完全二叉树的深度是log₂n向下取整加1。还有,如果一个完全二叉树的节点按层级编号,那么节点编号为i的节点的左子节点编号为2i,右子节点编号为2i+1。
通过这些基本概念和性质,我们可以构建出各种不同的二叉树,并针对它们执行不同的操作,如遍历、搜索、插入和删除。接下来的章节,我们将深入探讨二叉树的遍历理论基础,为后续的实践和高级技巧奠定基础。
# 2. 二叉树的遍历理论基础
## 2.1 遍历的定义与分类
### 2.1.1 遍历的定义
在计算机科学中,遍历是一种系统化访问数据结构中各个节点的过程,通常用于树和图等非线性数据结构。二叉树作为一种特殊的树形结构,其遍历过程尤为重要。遍历二叉树的基本目的是访问树中每一个节点一次且仅一次。在遍历过程中,我们根据访问节点的顺序,将遍历分为三种类型:前序遍历、中序遍历和后序遍历。通过这些遍历方式,我们能够以不同的方式获取树中数据的顺序,这对于树的搜索、排序和其他操作至关重要。
### 2.1.2 前序、中序、后序遍历的原理
- **前序遍历(Pre-order Traversal)**:在前序遍历中,我们首先访问根节点,然后递归地进行前序遍历右子树,接着进行前序遍历左子树。这种遍历方式的顺序可以概括为“根-右-左”。
- **中序遍历(In-order Traversal)**:中序遍历的特点是首先递归地进行中序遍历左子树,然后访问根节点,最后递归地进行中序遍历右子树。中序遍历的顺序是“左-根-右”。
- **后序遍历(Post-order Traversal)**:后序遍历先递归地进行后序遍历左子树,然后递归地进行后序遍历右子树,最后访问根节点。后序遍历的顺序是“左-右-根”。
这些遍历方式可以适用于所有类型的二叉树,并且可以用来打印树中的所有元素,或者用于复制树的结构等操作。下面我们将详细介绍每种遍历方式的原理,并探讨它们如何通过递归算法实现。
## 2.2 递归算法的原理
### 2.2.1 递归的定义与特性
递归是一种常见的编程技巧,它允许一个函数调用自身来解决问题。递归的基本思想是将一个问题分解成越来越小的相似子问题,直到达到一个简单的情况可以直接解决。对于二叉树遍历来说,递归允许我们按照“自顶向下”的策略,首先处理根节点,然后递归地处理子树。
递归有以下几个关键特性:
- **基准情形(Base Case)**:这是递归停止的条件,通常是一个最简单的情况,不需要再次递归调用。
- **递归情形(Recursive Case)**:这是函数调用自身以处理子问题的情况。
### 2.2.2 递归与栈的关系
尽管递归的实现非常直观,但它实际上是在使用系统栈来跟踪函数调用的状态。每次函数调用时,都会在栈上保存当前的状态(包括参数、局部变量等),当函数返回时,这些状态会从栈中弹出。因此,递归函数的每一次调用都会在栈上创建一个新的帧。
递归算法的时间复杂度分析往往与递归树的深度有关。例如,对于二叉树来说,其递归遍历的时间复杂度为O(n),其中n是树中节点的数量。这是因为每个节点恰好被访问一次。
下面我们将详细探讨二叉树遍历中递归算法的实现,以及如何编写和分析伪代码。
## 2.3 遍历的递归实现
### 2.3.1 递归遍历的伪代码分析
为了更好地理解递归遍历的工作原理,我们可以从伪代码开始。伪代码提供了一种简化的代码形式来表达算法的逻辑,而不依赖于具体的编程语言语法。
以下是前序遍历的递归伪代码示例:
```plaintext
PRE-ORDER(node):
if node is NULL:
return
visit(node)
PRE-ORDER(node.left)
PRE-ORDER(node.right)
```
中序遍历和后序遍历的伪代码遵循相同的逻辑结构,只是在访问节点的位置上有所不同。
### 2.3.2 递归算法的时间复杂度
递归算法的时间复杂度通常是根据递归调用的次数来确定的。对于二叉树遍历来说,每个节点被访问一次,因此时间复杂度是O(n),其中n是树中的节点数。需要注意的是,尽管时间复杂度是线性的,但是递归会消耗额外的空间复杂度,因为每次函数调用都会在栈上增加一个帧,其空间复杂度也是O(n)。
递归算法的性能还取决于递归树的深度,如果树非常不平衡,可能导致深度过大,从而增加调用栈的使用,并可能导致栈溢出错误。
现在我们已经对二叉树的递归遍历有了深入的理解,下面我们将通过具体的代码示例来演示如何实现递归遍历。
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def pre_order_traversal(root):
if root:
print(root.val, end=' ')
pre_order_traversal(root.left)
pre_order_traversal(root.right)
def in_order_traversal(root):
if root:
in_order_traversal(root.left)
print(root.val, end=' ')
in_order_traversal(root.right)
def post_order_traversal(root):
if root:
post_order_traversal(root.left)
post_order_traversal(root.right)
print(root.val, end=' ')
# 构建一个简单的测试树
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print("Pre-order traversal:")
pre_order_traversal(root)
print("\nIn-order traversal:")
in_order_traversal(root)
print("\nPost-order traversal:")
post_order_traversal(root)
```
在上述代码中,我们定义了一个简单的二叉树节点类`TreeNode`,以及三种遍历方法:`pre_order_traversal`、`in_order_traversal`和`post_order_traversal`。每种方法都遵循了先前伪代码描述的逻辑。我们还构建了一个小型的二叉树,并对其执行了三种遍历,打印出节点的值来展示遍历结果。
# 3. 二叉树遍历的递归实践
## 3.1 前序遍历的递归实现
在学习二叉树的前序遍历中,我们通常采用递归的策略。前序遍历的顺序是首先访问根节点,然后遍历左子树,最后遍历右子树。
### 3.1.1 递归代码编写
递归的实现依赖于一个简单的事实:任何一棵树的前序遍历可以看作是根节点加上其左子树的前序遍历和右子树的前序遍历的组合。
下面是一个典型的前序遍历递归函数的实现:
```python
class TreeNode:
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def preorderTraversal(root):
if not root:
return []
result = []
result.append(root.val) # 访问根节点
result += preorderTraversal(root.left) # 递归遍历左子树
result += preorderTraversal(root.right) # 递归遍历右子树
return result
```
### 3.1.2 递归遍历的实例演示
为了更直观地理解前序遍历的递归过程,
0
0
相关推荐
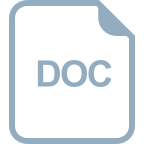
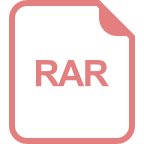
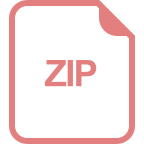





