Python去除列表里的逗号
时间: 2024-11-03 21:19:55 浏览: 4
在Python中,如果你想从列表中移除所有的逗号,你可以使用循环遍历结合字符串的replace()方法。这里是一个简单的例子:
```python
def remove_commas(lst):
# 创建一个新的空列表来保存处理后的元素
new_list = []
# 遍历原列表
for item in lst:
# 如果元素是字符串,替换其中的逗号为空
if isinstance(item, str):
new_item = item.replace(",", "")
else:
new_item = item # 如果不是字符串,则保持不变
# 将处理后的元素添加到新列表
new_list.append(new_item)
return new_list
# 示例用法
original_list = ['a, b', 'c', 'd, e,f']
new_list = remove_commas(original_list)
print(new_list) # 输出:['a b', 'c', 'd ef']
```
这个函数会返回一个新列表,其中原来的逗号都被删除了。
相关问题
python 去除逗号分隔
Python去除逗号分隔的方法有多种。一种常见的方法是使用字符串的replace()方法,将逗号替换为空字符串。另一种方法是使用split()方法将字符串按逗号分割成列表,然后再使用join()方法将列表中的元素连接成字符串。以下是两种方法的示例代码:
方法1: 使用replace()方法去除逗号分隔
```python
string = "apple,banana,orange"
result = string.replace(",", "")
print(result) # 输出: applebananaorange
```
方法2: 使用split()和join()方法去除逗号分隔
```python
string = "apple,banana,orange"
list1 = string.split(",")
result = "".join(list1)
print(result) # 输出: applebananaorange
```
python 字符串去除逗号
可以使用字符串的 replace 方法来去除逗号,具体方法如下:
```python
string = "1,2,3,4,5"
new_string = string.replace(",", "")
print(new_string)
```
输出结果为:
```
12345
```
在这个例子中,我们定义了一个字符串 `string`,其中包含了逗号。然后我们使用 `replace` 方法,将逗号替换为空字符串 `""`,从而去除了逗号。最后我们将新字符串输出到屏幕上。
阅读全文
相关推荐
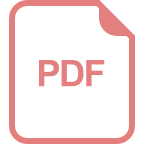
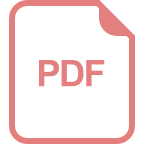
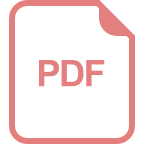













