输入一段英文串,去除其中的逗号和句号,再切成单词列表.
时间: 2023-05-11 09:04:18 浏览: 164
Sure, here's the code to achieve that:
```python
import re
text = "This is a sample text, with some commas and periods. Let's remove them!"
text = re.sub(r'[,.]', '', text) # remove commas and periods
words = text.split() # split into word list
print(words)
```
This code uses regular expressions to remove commas and periods from the input text, and then splits the resulting string into a list of words.
相关问题
用python语言输入一个字符串,除去其中四位逗号和句号,在切成单词列表。用replace()和split()方法
可以这样实现:
```python
string = "Hello, world! This is a test, string."
string = string.replace(",", "").replace(".", "") # 去除逗号和句号
word_list = string.split() # 切成单词列表
print(word_list)
```
输出结果为:
```
['Hello', 'world', 'This', 'is', 'a', 'test', 'string']
```
输入一段英文(标点符号只有叹号,问号,句号,逗号),找出所有单词,去除重复单词后(不区分大小写,如And和and是同一单词),按字典序排序输出所有单词。 提示:1)使用字符串的lower()方法将输入字符串全部转换为小写; 2)使用字符串的replace()方法把标点符号替换成空格,再使用split()切分单词 输入:一段英文 输出:每行一个单词 输入样例: The shortest way to do many things is to only one thing at a time. 输出样例: a at do is many one only shortest the thing things time to way
好的,针对你的问题,我可以给出以下 Python 代码实现:
```python
text = input().lower() # 读入一行英文并转换为小写
for punc in '.,?!': # 去除标点符号
text = text.replace(punc, ' ')
words = set(text.split()) # 切分单词并去重
for word in sorted(words): # 排序并输出单词
print(word)
```
这段代码先读入一行英文,并使用字符串的 `lower()` 方法将其全部转换为小写。然后使用字符串的 `replace()` 方法将标点符号替换为空格,并使用 `split()` 方法切分出单词列表。由于要去重并按字典序排序输出,可以将单词列表转换为集合,再使用 `sorted()` 方法排序,最后逐个输出。
阅读全文
相关推荐
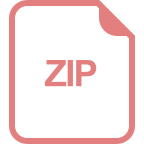
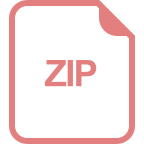












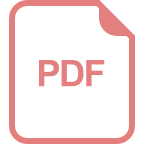