用C#通过url获取网页中的视频数据
时间: 2024-10-19 14:08:43 浏览: 44
在C#中,你可以使用HttpClient或者第三方库如AngleSharp来从URL获取网页中的视频数据。这里我会简单介绍如何使用HttpClient获取HTML源码,然后解析视频链接:
首先,确保已经安装了`System.Net.Http`命名空间,它是HttpClient的基础。
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class VideoDownloader
{
private readonly HttpClient _httpClient = new HttpClient();
public async Task<string> GetWebPageContent(string url)
{
try
{
HttpResponseMessage response = await _httpClient.GetAsync(url);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
catch (HttpRequestException ex)
{
Console.WriteLine($"Error fetching URL: {ex.Message}");
return null;
}
}
// 这里只是一个基础示例,实际解析HTML找到视频链接需要更复杂的方法
public string FindVideoLinkFromHtml(string html)
{
// 使用正则表达式、HTML Agility Pack或其他库来搜索video标签或iframe src属性
// 这部分你需要自己实现,因为这取决于目标网站的具体结构
throw new NotImplementedException("To find video links from HTML, implement a parser for the specific website structure.");
}
}
// 调用方法获取内容并解析视频链接
string url = "https://example.com";
string htmlContent = await new VideoDownloader().GetWebPageContent(url);
string videoLink = await new VideoDownloader().FindVideoLinkFromHtml(htmlContent);
```
请注意,上述代码只是基本示例,并未包含完整的HTML解析功能。在实际应用中,你可能需要处理各种情况,比如使用 AngleSharp 解析DOM树,或者针对不同的视频格式和嵌入方式调整策略。
阅读全文
相关推荐
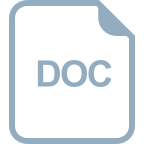
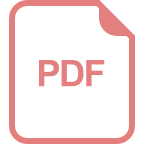
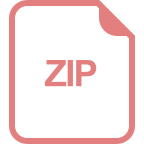















