输入一个英文字母(可能是大写,也可能是小写),输出该字母在字母表中的序号(’a’和’A’的序号为1)。
时间: 2024-09-27 16:09:46 浏览: 122
在C语言中,可以编写一个函数来计算输入英文字母的序号。这里我们只考虑单个字母的情况,并忽略其他字符。我们可以利用ASCII码的特性,因为大写字母和小写字母在ASCII表中的顺序是连续的,所以只需判断字母的大、小写状态再加相应的偏移量即可。
```c
#include <stdio.h>
int get_letter_index(char letter) {
if ('a' <= letter && letter <= 'z') { // 小写字母
return letter - 'a' + 1;
} else if ('A' <= letter && letter <= 'Z') { // 大写字母
return letter - 'A' + 1;
} else {
printf("输入不是字母!\n");
return -1; // 或者抛出异常,取决于你的需求
}
}
int main() {
char input;
printf("请输入一个字母(区分大小写): ");
scanf("%c", &input);
int index = get_letter_index(input);
if (index != -1) {
printf("字母 '%c' 的序号是 %d.\n", input, index);
}
return 0;
}
```
相关问题
用 从键盘输入一个英文字母在英文字母表中的序号,然后输出相对应的这个字母的大写和小写
字母。
以下是Python的实现代码:
```python
letter = input("请输入一个英文字母:")
# 将输入的字母转换为小写字母
letter = letter.lower()
# 计算字母在字母表中的序号
index = ord(letter) - ord('a') + 1
# 输出相应的大写和小写字母
print("相应的大写字母是:", chr(index + ord('A') - 1))
print("相应的小写字母是:", chr(index + ord('a') - 1))
```
运行程序时,输入一个英文字母,程序会输出相应的大写和小写字母。例如,输入字母"b",程序会输出:
```
相应的大写字母是: B
相应的小写字母是: b
```
用c语言写出输入一个大写字母,请输出它的小写字母,以及这个它在字母表中的序号的代码
#include <stdio.h>int main()
{
char ch;
printf("请输入一个大写字母:");
scanf("%c", &ch);
char lower_ch = ch + 32;
int order = ch - 'A' + 1;
printf("小写字母为:%c\n", lower_ch);
printf("字母表中的序号为:%d\n", order);
return 0;
}
阅读全文
相关推荐
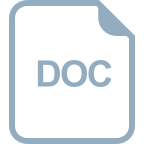
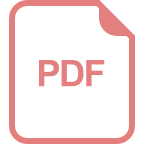












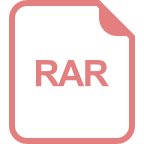
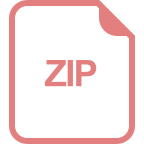