用c语言写操作系统中的生产者与消费者问题
时间: 2024-10-27 11:05:15 浏览: 5
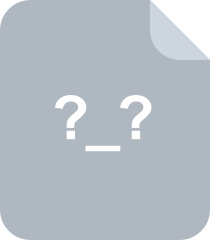
C语言实现操作系统中生产者消费者
在C语言中,生产者-消费者问题是一个经典的并发编程示例,它模拟了两个线程之间的通信,一个是生产者(Producer),负责生成数据放入缓冲区;另一个是消费者(Consumer),从缓冲区取出数据进行处理。通常会借助队列(如数组或链表)作为共享资源。
下面是一个简单的C语言版本的生产者-消费者模型:
```c
#include <stdio.h>
#include <stdlib.h>
#include <semaphore.h> // 使用信号量库
#define MAX_QUEUE_SIZE 10
typedef struct {
int data[MAX_QUEUE_SIZE];
int front, rear;
} Queue;
// 生产者函数
void producer(Queue* queue, int count) {
sem_t empty = 0; // 空闲信号量
sem_t full = MAX_QUEUE_SIZE - 1; // 满载信号量
for (int i = 0; i < count; i++) {
sem_wait(&empty); // 当缓冲区为空时等待
queue->data[queue->rear] = i; // 插入元素
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
sem_post(&full); // 缓冲区满,唤醒消费者
printf("Produced item %d\n", i);
}
}
// 消费者函数
void consumer(Queue* queue) {
sem_t empty = 0;
sem_t full = MAX_QUEUE_SIZE - 1;
while (1) {
sem_wait(&full); // 当缓冲区非空时等待
if (queue->front == queue->rear) { // 如果队列已空,则退出循环
break;
}
int item = queue->data[queue->front]; // 取出元素
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
sem_post(&empty); // 缓冲区不满,通知生产者可以继续
printf("Consumed item %d\n", item);
}
}
int main() {
Queue queue;
sem_init(&empty, 0, 0);
sem_init(&full, 0, MAX_QUEUE_SIZE);
// 创建生产者和消费者线程
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, &queue);
pthread_create(&consumer_thread, NULL, consumer, &queue);
// 等待所有线程完成
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
阅读全文
相关推荐
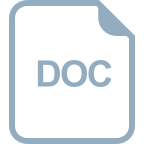
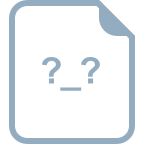

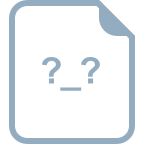
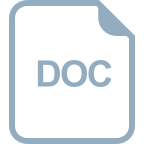
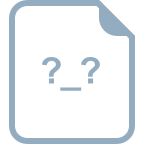
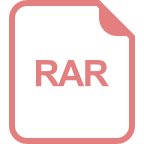
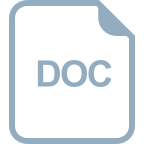
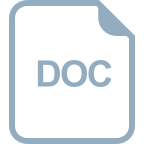








