for (const Flight& flight : flights)怎么更改可以不用C++11
时间: 2023-12-13 16:04:14 浏览: 36
你可以使用传统的循环来替代范围-based for循环,如下所示:
```cpp
for (std::vector<Flight>::const_iterator it = flights.begin(); it != flights.end(); ++it) {
const Flight& flight = *it;
// 在这里进行你的操作
}
```
这里使用了一个迭代器 `it` 来遍历 `flights` 向量中的每个元素,并将其赋值给 `flight`。你可以在循环体内进行你的操作,就像在范围-based for循环中一样。要注意的是,`const_iterator` 用于确保不会更改 `flights` 中的元素。
相关问题
请用C++stl航空管理系统
好的,以下是一个简单的航空管理系统的实现,使用了C++的STL库:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
class Flight {
public:
Flight(string id, string from, string to, int seats) : m_id(id), m_from(from), m_to(to), m_seats(seats) {}
string getID() const { return m_id; }
string getFrom() const { return m_from; }
string getTo() const { return m_to; }
int getSeats() const { return m_seats; }
private:
string m_id;
string m_from;
string m_to;
int m_seats;
};
class Airline {
public:
Airline(string name) : m_name(name) {}
string getName() const { return m_name; }
const vector<Flight>& getFlights() const { return m_flights; }
void addFlight(const Flight& flight) { m_flights.push_back(flight); }
private:
string m_name;
vector<Flight> m_flights;
};
class Airport {
public:
void addAirline(const Airline& airline) { m_airlines.push_back(airline); }
const vector<Airline>& getAirlines() const { return m_airlines; }
private:
vector<Airline> m_airlines;
};
int main() {
Airport airport;
// 添加航空公司和航班信息
Airline airline1("Airline 1");
airline1.addFlight(Flight("001", "Beijing", "Shanghai", 200));
airline1.addFlight(Flight("002", "Beijing", "Guangzhou", 150));
airline1.addFlight(Flight("003", "Shanghai", "Guangzhou", 180));
airline1.addFlight(Flight("004", "Shanghai", "Beijing", 200));
airport.addAirline(airline1);
Airline airline2("Airline 2");
airline2.addFlight(Flight("101", "Beijing", "Shanghai", 250));
airline2.addFlight(Flight("102", "Beijing", "Guangzhou", 200));
airline2.addFlight(Flight("103", "Shanghai", "Guangzhou", 220));
airline2.addFlight(Flight("104", "Shanghai", "Beijing", 250));
airport.addAirline(airline2);
// 查询航班信息
string from = "Beijing";
string to = "Guangzhou";
for (const Airline& airline : airport.getAirlines()) {
for (const Flight& flight : airline.getFlights()) {
if (flight.getFrom() == from && flight.getTo() == to) {
cout << airline.getName() << " " << flight.getID() << " " << flight.getSeats() << endl;
}
}
}
// 排序航班信息
vector<Flight> allFlights;
for (const Airline& airline : airport.getAirlines()) {
allFlights.insert(allFlights.end(), airline.getFlights().begin(), airline.getFlights().end());
}
sort(allFlights.begin(), allFlights.end(), [](const Flight& a, const Flight& b) { return a.getSeats() < b.getSeats(); });
for (const Flight& flight : allFlights) {
cout << flight.getID() << " " << flight.getFrom() << " " << flight.getTo() << " " << flight.getSeats() << endl;
}
return 0;
}
```
这个系统包含三个类:Flight(航班信息)、Airline(航空公司)和Airport(机场)。一个机场可以拥有多个航空公司,每个航空公司可以有多个航班。
在这个简单的示例中,我们添加了两个航空公司,每个航空公司有四个航班。我们还演示了如何查询从北京到广州的航班信息,以及如何按航班座位数排序所有航班信息。
航空客运订票系统c++
航空客运订票系统的设计与实现是一个可以实现航空客运订票的系统。它包括了浏览和查询航班信息、机票预订和办理退票等主要业务活动。以下是一个使用C++实现的简单示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Flight {
string start;
string destination;
string number;
string airplaneNumber;
string flightDate;
int totalSeats;
int availableSeats;
int firstClassSeats;
int businessClassSeats;
int economyClassSeats;
double firstClassPrice;
double businessClassPrice;
double economyClassPrice;
};
vector<Flight> flights;
void addFlight() {
Flight flight;
cout << "Enter start: ";
cin >> flight.start;
cout << "Enter destination: ";
cin >> flight.destination;
cout << "Enter number: ";
cin >> flight.number;
cout << "Enter airplane number: ";
cin >> flight.airplaneNumber;
cout << "Enter flight date: ";
cin >> flight.flightDate;
cout << "Enter total seats: ";
cin >> flight.totalSeats;
cout << "Enter available seats: ";
cin >> flight.availableSeats;
cout << "Enter first class seats: ";
cin >> flight.firstClassSeats;
cout << "Enter business class seats: ";
cin >> flight.businessClassSeats;
cout << "Enter economy class seats: ";
cin >> flight.economyClassSeats;
cout << "Enter first class price: ";
cin >> flight.firstClassPrice;
cout << "Enter business class price: ";
cin >> flight.businessClassPrice;
cout << "Enter economy class price: ";
cin >> flight.economyClassPrice;
flights.push_back(flight);
cout << "Flight added successfully!" << endl;
}
void deleteFlight() {
string number;
cout << "Enter flight number to delete: ";
cin >> number;
for (int i = 0; i < flights.size(); i++) {
if (flights[i].number == number) {
flights.erase(flights.begin() + i);
cout << "Flight deleted successfully!" << endl;
return;
}
}
cout << "Flight not found!" << endl;
}
void displayFlights() {
for (const Flight& flight : flights) {
cout << "Start: " << flight.start << endl;
cout << "Destination: " << flight.destination << endl;
cout << "Number: " << flight.number << endl;
cout << "Airplane Number: " << flight.airplaneNumber << endl;
cout << "Flight Date: " << flight.flightDate << endl;
cout << "Total Seats: " << flight.totalSeats << endl;
cout << "Available Seats: " << flight.availableSeats << endl;
cout << "First Class Seats: " << flight.firstClassSeats << endl;
cout << "Business Class Seats: " << flight.businessClassSeats << endl;
cout << "Economy Class Seats: " << flight.economyClassSeats << endl;
cout << "First Class Price: " << flight.firstClassPrice << endl;
cout << "Business Class Price: " << flight.businessClassPrice << endl;
cout << "Economy Class Price: " << flight.economyClassPrice << endl;
cout << endl;
}
}
int main() {
int choice;
do {
cout << "1. Add Flight" << endl;
cout << "2. Delete Flight" << endl;
cout << "3. Display Flights" << endl;
cout << "0. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addFlight();
break;
case 2:
deleteFlight();
break;
case 3:
displayFlights();
break;
case 0:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice!" << endl;
break;
}
cout << endl;
} while (choice != 0);
return 0;
}
```
这个示例实现了航空客运订票系统的基本功能,包括添加航班、删除航班和显示航班信息。你可以根据自己的需求进行扩展和修改。
相关推荐
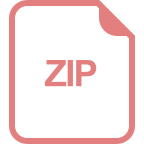
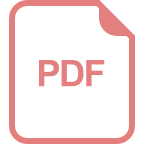
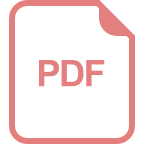
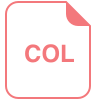
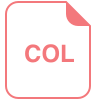
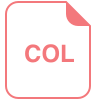
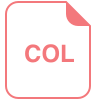









