slowfast与LSTM结合代码
时间: 2023-07-24 21:02:38 浏览: 64
以下是将 LSTM 结合到 SlowFast 模型中的代码示例:
```python
import torch
import torch.nn as nn
class SlowFastLSTM(nn.Module):
def __init__(self):
super(SlowFastLSTM, self).__init__()
# Slow pathway
self.slow_conv = nn.Conv3d(in_channels=3, out_channels=64, kernel_size=5, stride=1, padding=2)
self.slow_lstm = nn.LSTM(input_size=64, hidden_size=128, num_layers=2, batch_first=True)
# Fast pathway
self.fast_conv = nn.Conv3d(in_channels=3, out_channels=8, kernel_size=1, stride=1)
self.fast_lstm = nn.LSTM(input_size=8, hidden_size=32, num_layers=1, batch_first=True)
# Fusion
self.fusion_linear = nn.Linear(128 + 32, 256)
def forward(self, x):
# Slow pathway
slow_out = self.slow_conv(x)
b, c, t, h, w = slow_out.size()
slow_out = slow_out.view(b, c, t * h * w).permute(0, 2, 1) # Reshape for LSTM input: (batch_size, sequence_length, input_size)
_, (slow_hidden, _) = self.slow_lstm(slow_out)
# Fast pathway
fast_out = self.fast_conv(x)
b, c, t, h, w = fast_out.size()
fast_out = fast_out.view(b, c, t * h * w).permute(0, 2, 1) # Reshape for LSTM input: (batch_size, sequence_length, input_size)
_, (fast_hidden, _) = self.fast_lstm(fast_out)
# Fusion
fusion_out = torch.cat((slow_hidden[-1], fast_hidden[-1]), dim=1) # Concatenate the last hidden states from both pathways
fusion_out = self.fusion_linear(fusion_out)
return fusion_out
```
在上述代码中,`SlowFastLSTM` 类定义了将 LSTM 结合到 SlowFast 模型中的网络结构。模型包括 Slow 和 Fast 路径的卷积层,以及相应的 LSTM 层。在 `forward` 方法中,首先将输入数据通过 Slow 和 Fast 路径的卷积层,并对输出进行多维度的重排和转置,以适应 LSTM 的输入格式。然后,将重排后的数据输入到相应的 LSTM 层中进行时序建模。最后,将 Slow 和 Fast 路径 LSTM 的最后一个隐藏状态连接起来,并通过线性层进行融合。
请注意,上述代码仅为示例,具体的模型架构和参数设置可能需要根据具体任务进行调整和优化。此外,还可以根据实际需求添加其他层或模块,如注意力机制等,来进一步改进模型性能。
相关推荐
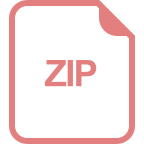














