数据结构c语言重要习题
时间: 2023-12-12 15:34:41 浏览: 108
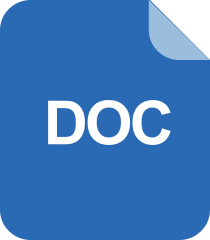
C语言数据结构习题,习题
以下是几个数据结构C语言的重要习题:
1. 实现一个栈数据结构
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int value) {
if (is_full(s)) {
printf("Stack is full.\n");
return;
}
s->data[++s->top] = value;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty.\n");
return -1;
}
return s->data[s->top--];
}
int main() {
Stack s;
init(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
printf("%d\n", pop(&s)); // 输出:3
printf("%d\n", pop(&s)); // 输出:2
printf("%d\n", pop(&s)); // 输出:1
printf("%d\n", pop(&s)); // 输出:Stack is empty. -1
return 0;
}
```
2. 实现一个队列数据结构
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int front;
int rear;
} Queue;
void init(Queue *q) {
q->front = q->rear = 0;
}
int is_empty(Queue *q) {
return q->front == q->rear;
}
int is_full(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
void enqueue(Queue *q, int value) {
if (is_full(q)) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = value;
q->rear = (q->rear + 1) % MAX_SIZE;
}
int dequeue(Queue *q) {
if (is_empty(q)) {
printf("Queue is empty.\n");
return -1;
}
int value = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return value;
}
int main() {
Queue q;
init(&q);
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
printf("%d\n", dequeue(&q)); // 输出:1
printf("%d\n", dequeue(&q)); // 输出:2
printf("%d\n", dequeue(&q)); // 输出:3
printf("%d\n", dequeue(&q)); // 输出:Queue is empty. -1
return 0;
}
```
3. 实现一个链表数据结构
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *create_node(int value) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = value;
node->next = NULL;
return node;
}
void insert(Node **head, int value) {
Node *node = create_node(value);
node->next = *head;
*head = node;
}
void delete(Node **head, int value) {
Node *prev = NULL;
Node *curr = *head;
while (curr != NULL && curr->data ! value) {
prev = curr;
curr = curr->next;
}
if (curr == NULL) {
printf("Value not found.\n");
return;
}
if (prev == NULL) {
*head = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
}
void print_list(Node *head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
Node *head = NULL;
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
print_list(head); // 输出:3 2 1
delete(&head, 2);
print_list(head); // 输出:3 1
delete(&head, 4); // 输出:Value not found.
return 0;
}
```
阅读全文
相关推荐
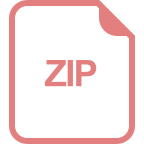
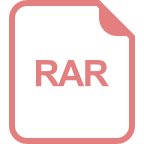
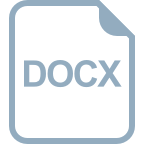
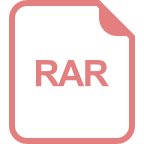
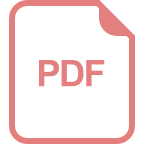
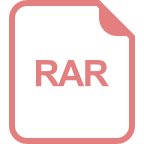