C语言银行排队系统问题
时间: 2024-03-16 08:37:45 浏览: 208
银行排队系统是一个常见的仿真问题,可以用C语言实现。以下是一个基本的单队列、多窗口的银行排队系统的实现,包括客户进入排队系统、客户离开、查询当前客户前面还有几人、查询截至目前总共办理多少客户等功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_QUEUE_SIZE 100 // 队列最大长度
#define MAX_WINDOW_NUM 10 // 窗口最大数量
typedef struct {
int id; // 客户编号
int type; // 客户类型,0表示普通客户,1表示VIP客户
int arrive_time; // 到达时间
int service_time; // 服务时间
int start_time; // 开始服务时间
int end_time; // 结束服务时间
} Customer;
typedef struct {
Customer data[MAX_QUEUE_SIZE]; // 队列数据
int front; // 队头指针
int rear; // 队尾指针
} Queue;
typedef struct {
int id; // 窗口编号
int type; // 窗口类型,0表示普通窗口,1表示VIP窗口
int busy; // 窗口是否忙碌,0表示空闲,1表示忙碌
int start_time; // 开始服务时间
int end_time; // 结束服务时间
} Window;
int customer_id = 0; // 客户编号计数器
int total_customer_num = 0; // 总共办理客户数量
int total_wait_time = 0; // 总共等待时间
int total_service_time = 0; // 总共服务时间
int window_num = 0; // 窗口数量
Window windows[MAX_WINDOW_NUM]; // 窗口数组
Queue queue; // 队列
// 初始化队列
void init_queue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int is_queue_empty(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int is_queue_full(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
// 入队
void enqueue(Queue *q, Customer c) {
if (is_queue_full(q)) {
printf("Queue is full!\n");
return;
}
q->data[q->rear] = c;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
// 出队
Customer dequeue(Queue *q) {
if (is_queue_empty(q)) {
printf("Queue is empty!\n");
exit(1);
}
Customer c = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return c;
}
// 初始化窗口
void init_windows() {
for (int i = 0; i < window_num; i++) {
windows[i].id = i + 1;
windows[i].type = 0;
windows[i].busy = 0;
windows[i].start_time = 0;
windows[i].end_time = 0;
}
}
// 获取空闲窗口
int get_idle_window() {
for (int i = 0; i < window_num; i++) {
if (windows[i].busy == 0) {
return i;
}
}
return -1;
}
// 获取最早结束服务的窗口
int get_earliest_window() {
int earliest = 0;
for (int i = 1; i < window_num; i++) {
if (windows[i].end_time < windows[earliest].end_time) {
earliest = i;
}
}
return earliest;
}
// 获取VIP窗口
int get_vip_window() {
for (int i = 0; i < window_num; i++) {
if (windows[i].type == 1 && windows[i].busy == 0) {
return i;
}
}
return -1;
}
// 生成随机数
int random_int(int min, int max) {
return rand() % (max - min + 1) + min;
}
// 生成客户
Customer generate_customer(int arrive_time) {
Customer c;
c.id = ++customer_id;
c.type = random_int(0, 1);
c.arrive_time = arrive_time;
c.service_time = random_int(1, 10);
c.start_time = 0;
c.end_time = 0;
return c;
}
// 处理客户
void process_customer(Customer c) {
int idle_window = get_idle_window();
if (idle_window != -1) { // 有空闲窗口
windows[idle_window].busy = 1;
windows[idle_window].type = c.type;
windows[idle_window].start_time = c.arrive_time;
windows[idle_window].end_time = c.arrive_time + c.service_time;
c.start_time = windows[idle_window].start_time;
c.end_time = windows[idle_window].end_time;
total_service_time += c.service_time;
total_wait_time += c.start_time - c.arrive_time;
} else { // 没有空闲窗口,加入队列
enqueue(&queue, c);
}
}
// 处理窗口
void process_window(int current_time) {
for (int i = 0; i < window_num; i++) {
if (windows[i].busy && windows[i].end_time == current_time) { // 窗口服务结束
windows[i].busy = 0;
total_customer_num++;
if (!is_queue_empty(&queue)) { // 队列不为空,取出队首客户进行服务
Customer c = dequeue(&queue);
windows[i].busy = 1;
windows[i].type = c.type;
windows[i].start_time = current_time;
windows[i].end_time = current_time + c.service_time;
c.start_time = windows[i].start_time;
c.end_time = windows[i].end_time;
total_service_time += c.service_time;
total_wait_time += c.start_time - c.arrive_time;
}
}
}
}
// 打印客户信息
void print_customer(Customer c) {
printf("Customer %d: type=%d, arrive_time=%d, service_time=%d, start_time=%d, end_time=%d\n",
c.id, c.type, c.arrive_time, c.service_time, c.start_time, c.end_time);
}
// 打印窗口信息
void print_window(Window w) {
printf("Window %d: type=%d, busy=%d, start_time=%d, end_time=%d\n",
w.id, w.type, w.busy, w.start_time, w.end_time);
}
// 打印客户队列信息
void print_queue(Queue *q) {
printf("Queue: ");
for (int i = q->front; i != q->rear; i = (i + 1) % MAX_QUEUE_SIZE) {
printf("%d ", q->data[i].id);
}
printf("\n");
}
// 打印窗口信息
void print_windows() {
for (int i = 0; i < window_num; i++) {
print_window(windows[i]);
}
}
// 打印排队系统信息
void print_system(int current_time) {
printf("Current time: %d\n", current_time);
printf("Total customer num: %d\n", total_customer_num);
printf("Total wait time: %d\n", total_wait_time);
printf("Total service time: %d\n", total_service_time);
printf("Average wait time: %.2f\n", (float) total_wait_time / total_customer_num);
printf("Window status:\n");
print_windows();
printf("Queue status:\n");
print_queue(&queue);
}
int main() {
srand(time(NULL));
printf("Please input the number of windows: ");
scanf("%d", &window_num);
init_windows();
init_queue(&queue);
int current_time = 0;
while (current_time < 480) { // 一天的运行时间为480分钟
Customer c = generate_customer(current_time);
printf("Customer %d arrives at %d, type=%d, service_time=%d\n",
c.id, c.arrive_time, c.type, c.service_time);
process_customer(c);
print_system(current_time);
process_window(current_time);
current_time++;
}
printf("Simulation ends.\n");
return 0;
}
```
阅读全文
相关推荐
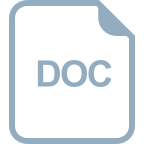
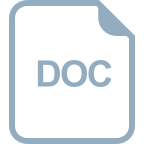
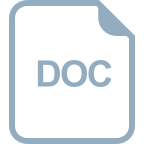











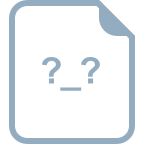
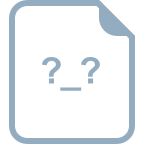