你帮我编写一个最简单的 python a*船舶路径规划代码
时间: 2024-09-07 21:04:24 浏览: 30
A*算法是一种启发式搜索算法,常用于路径规划问题。在编写最简单的Python A*路径规划代码时,我们需要定义几个关键部分:节点表示、启发函数计算、节点选择和路径回溯。下面是一个简化版的A*算法实现,用于船舶路径规划:
```python
import heapq
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 # 从起始点到当前点的成本
self.h = 0 # 当前点到目标点的估计成本(启发式)
self.f = 0 # 总成本
def __lt__(self, other):
return self.f < other.f
def heuristic(start, end):
# 使用曼哈顿距离作为启发式函数
return abs(start[0] - end[0]) + abs(start[1] - end[1])
def astar(start, end, grid):
open_list = []
closed_list = set()
start_node = Node(start)
end_node = Node(end)
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node.position)
if current_node.position == end_node.position:
return reconstruct_path(current_node)
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]: # 上下左右移动
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if node_position in closed_list:
continue
if not is_valid(node_position, grid):
continue
new_node = Node(node_position, current_node)
new_node.g = current_node.g + 1
new_node.h = heuristic(new_node.position, end_node.position)
new_node.f = new_node.g + new_node.h
if add_to_open(open_list, new_node):
heapq.heappush(open_list, new_node)
return None
def reconstruct_path(node):
path = []
while node:
path.append(node.position)
node = node.parent
return path[::-1] # 反转路径
def is_valid(position, grid):
# 这里需要自定义逻辑判断位置是否有效
# 例如,可以检查网格上该位置是否为可通行
return True
def add_to_open(open_list, node):
for n in open_list:
if n == node and n.g >= node.g:
return False
return True
# 使用示例
start = (0, 0) # 起始点坐标
end = (3, 3) # 目标点坐标
grid = [[0 for _ in range(4)] for _ in range(4)] # 定义一个4x4的网格,0表示可通行
path = astar(start, end, grid)
print(path)
```
请注意,上述代码中的`is_valid`函数需要根据实际情况进行定义,以确保节点有效。另外,网格大小、起始点和结束点的位置以及船舶的移动方向(上下左右移动)都可以根据实际情况进行调整。
阅读全文
相关推荐
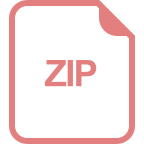
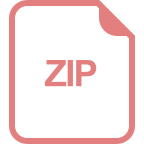
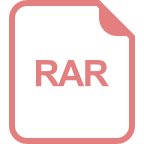















