C++定义一个人员类person为虚基类,包含人员编号和姓名以及相关的成员函数。从它派生出教师类teacher和医生类doctor。然后再从这两个类派生出教师医生类techdoc。 其中,教师类包含系别和职称。医生类包含科室和研究专业。
时间: 2024-12-22 08:12:19 浏览: 8
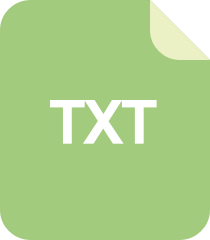
设计一个人类person,包括人员编号和姓名等数据成员以及相关的成员函数
在C++中,你可以通过以下步骤定义一个基于虚基类`Person`的结构,然后分别派生出`Teacher`、`Doctor`和`TechDoc`类:
首先,我们创建一个虚基类`Person`,包含基础属性如编号(`id`)和姓名(`name`),以及虚函数:
```cpp
// Person.h
class Person {
public:
virtual ~Person() {} // 虚析构函数
int id;
std::string name;
protected:
// 使用virtual关键字声明虚基类,使得派生类的构造函数自动调用基类的构造函数
Person(int id, const std::string& name) : id(id), name(name) {}
// 公开一些虚函数,例如获取信息的函数
virtual std::string getRole() = 0; // 纯虚函数,子类需要覆盖
};
```
接下来,我们定义`Teacher`和`Doctor`类作为`Person`的派生类,并添加各自的特性:
```cpp
// Teacher.h
class Teacher : public Person {
public:
Teacher(int id, const std::string& name, const std::string& department, const std::string& title)
: Person(id, name), department(department), title(title) {}
std::string getRole() override { return "Teacher"; }
std::string getDepartment() const { return department; }
std::string getTitle() const { return title; }
private:
std::string department;
std::string title;
};
// Doctor.h
class Doctor : public Person {
public:
Doctor(int id, const std::string& name, const std::string& specialty, const std::string& research)
: Person(id, name), specialty(specialty), research(research) {}
std::string getRole() override { return "Doctor"; }
std::string getSpecialty() const { return specialty; }
std::string getResearch() const { return research; }
private:
std::string specialty;
std::string research;
};
```
最后,定义`TechDoc`类,它是`Teacher`和`Doctor`的双重衍生类:
```cpp
// TechDoc.h
class TechDoc : public Teacher, public Doctor {
public:
TechDoc(int id, const std::string& name, const std::string& department, const std::string& title,
const std::string& specialty, const std::string& research)
: Teacher(id, name, department, title), Doctor(id, name, specialty, research) {}
std::string getRole() override {
return "TechDoc";
}
};
```
阅读全文
相关推荐
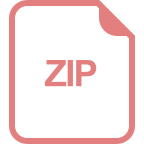
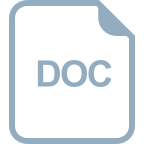
















