用c++输入10个国家名称,用指针数组实现排序输出。
时间: 2024-10-13 15:17:56 浏览: 28
在C++中,你可以使用`cin`来获取用户输入的国家名称,并通过指针数组存储它们。然后可以利用标准库中的`sort`函数对字符串指针数组进行排序。下面是一个简单的示例:
```cpp
#include <iostream>
#include <algorithm> // 包含sort函数
using namespace std;
// 定义结构体存放国家名及其指针
struct Country {
string name;
char* namePtr; // 指向名字的字符指针
};
int main() {
const int size = 10;
Country countries[size]; // 创建指针数组
for (int i = 0; i < size; ++i) {
cout << "请输入第" << i + 1 << "个国家的名称:";
cin >> countries[i].name; // 输入并保存国家名称
countries[i].namePtr = new char[countries[i].name.size() + 1];
strcpy(countries[i].namePtr, countries[i].name.c_str()); // 将名字复制到指针指向的内存
}
// 对国家名称指针数组进行排序
sort(countries, countries + size, [](const Country& a, const Country& b) { return strcmp(a.namePtr, b.namePtr) < 0; });
// 输出排序后的结果
for (int i = 0; i < size; ++i) {
cout << "排序后:" << countries[i].name << endl;
}
// 清理内存
for (Country& c : countries) {
delete[] c.namePtr;
}
return 0;
}
阅读全文
相关推荐
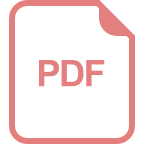
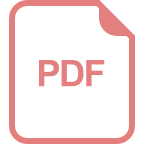
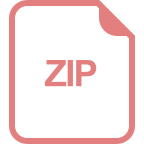















