AttributeError: 'Model' object has no attribute 'test'
时间: 2024-05-20 19:09:31 浏览: 17
这个错误是因为你在一个Python类的实例上调用了一个不存在的属性或方法。具体来说,在你的代码中,你调用了一个名为“test”的属性或方法,但是该属性或方法在类定义中没有被定义。可能的原因包括:
- 你打错了属性或方法的名称。
- 你忘记定义该属性或方法。
- 你在子类中尝试调用父类中不存在的属性或方法。
解决此错误的方法是检查你的代码并确保你引用的属性或方法确实存在,并且正确地使用了它。如果你不确定如何解决这个问题,可以提供更多代码上下文和详细信息,以便我能够更好地帮助你。
相关问题
AttributeError: 'Sequential' object has no attribute 'model'
根据提供的引用内容,报错信息显示`AttributeError: 'Sequential' object has no attribute 'predict_classes'`,这意味着在使用`Sequential`对象时,没有名为`predict_classes`的属性。
在TensorFlow 2.0及以上版本中,`predict_classes`方法已被弃用。相反,您可以使用`predict`方法来获取预测的类别。下面是一个示例代码:
```python
# 导入必要的库
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# 创建模型
model = Sequential()
model.add(Dense(10, input_shape=(10,), activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# 编译模型
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=10, batch_size=32)
# 使用predict方法进行预测
predictions = model.predict(x_test[0:10])
predicted_classes = tf.argmax(predictions, axis=1)
# 打印预测结果
print(predicted_classes)
```
请注意,上述代码仅为示例,您需要根据您的模型和数据进行相应的修改。
AttributeError: 'Model' object has no attribute 'hidden_size'
对不起,我之前的回答中有一个错误。抱歉给你带来了困惑。
由于 `hidden_size` 参数是在模型类的 `__init__` 方法中定义的,所以无法直接通过修改 `model.hidden_size` 来改变模型的隐藏层大小。相反,我们需要重新创建一个新的模型对象,并将新的隐藏层大小作为参数传递给模型。
以下是修改后的示例代码:
```python
import torch
import torch.nn as nn
from sklearn.model_selection import ParameterGrid
from sklearn.datasets import load_iris
from sklearn.metrics import accuracy_score
# 加载数据集
data = load_iris()
X_train = data.data[:100]
y_train = data.target[:100]
X_test = data.data[100:]
y_test = data.target[100:]
# 定义模型
class Model(nn.Module):
def __init__(self, hidden_size):
super(Model, self).__init__()
self.fc = nn.Linear(4, hidden_size)
self.relu = nn.ReLU()
self.output = nn.Linear(hidden_size, 3)
def forward(self, x):
x = self.fc(x)
x = self.relu(x)
x = self.output(x)
return x
# 定义超参数的网格
param_grid = {
'hidden_size': [32, 64, 128],
'lr': [0.001, 0.01, 0.1]
}
# 遍历超参数网格
best_score = 0
best_params = None
for params in ParameterGrid(param_grid):
# 创建模型实例
model = Model(hidden_size=params['hidden_size'])
# 在训练集上训练模型
optimizer = torch.optim.SGD(model.parameters(), lr=params['lr'])
criterion = nn.CrossEntropyLoss()
for epoch in range(num_epochs):
# 前向传播和反向传播
# ...
# 在测试集上进行预测
model.eval()
with torch.no_grad():
y_pred = model(torch.Tensor(X_test))
y_pred = torch.argmax(y_pred, dim=1).numpy()
# 计算模型在测试集上的准确率
score = accuracy_score(y_test, y_pred)
# 更新最佳得分和最佳参数
if score > best_score:
best_score = score
best_params = params
# 输出最佳参数和得分
print("最佳参数:", best_params)
print("最佳得分:", best_score)
```
在这个修改后的代码中,我们在每个参数组合的循环中创建了一个新的模型实例,并将 `hidden_size` 参数作为参数传递给模型。这样,我们就可以根据不同的参数组合创建不同大小的隐藏层。
非常抱歉给你带来了困扰,现在你可以使用修改后的代码来进行模型的超参数网格搜索。如果还有其他问题,请随时提问。
相关推荐
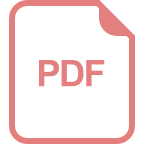
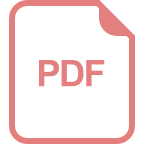
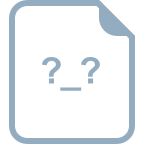












