c++复数的四则运算
时间: 2023-12-06 16:36:36 浏览: 100
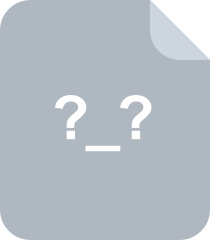
c++用复数实现四则运算
C++中的复数四则运算可以通过自定义复数类来实现。下面是一个复数类的示例代码,其中包括了加、减、乘、除四种运算:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& c2) const { // 加法运算
return Complex(real + c2.real, imag + c2.imag);
}
Complex operator-(const Complex& c2) const { // 减法运算
return Complex(real - c2.real, imag - c2.imag);
}
Complex operator*(const Complex& c2) const { // 乘法运算
return Complex(real * c2.real - imag * c2.imag, real * c2.imag + imag * c2.real);
}
Complex operator/(const Complex& c2) const { // 除法运算
double denominator = c2.real * c2.real + c2.imag * c2.imag;
return Complex((real * c2.real + imag * c2.imag) / denominator, (imag * c2.real - real * c2.imag) / denominator);
}
bool operator==(const Complex& c2) const { // 判断两个复数是否相等
return (real == c2.real) && (imag == c2.imag);
}
bool operator!=(const Complex& c2) const { // 判断两个复数是否不相等
return !(*this == c2);
}
friend ostream& operator<<(ostream& os, const Complex& c); // 重载输出运算符
};
ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
int main() {
Complex a(1, 2);
Complex b(3, 4);
Complex c = a + b;
Complex d = a - b;
Complex e = a * b;
Complex f = a / b;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a + b = " << c << endl;
cout << "a - b = " << d << endl;
cout << "a * b = " << e << endl;
cout << "a / b = " << f << endl;
if (a == b) {
cout << "a == b" << endl;
}
if (a != b) {
cout << "a != b" << endl;
}
return 0;
}
```
阅读全文
相关推荐
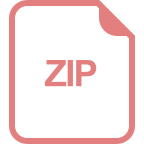
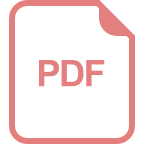
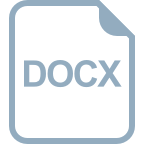
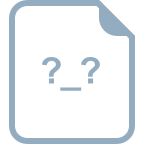
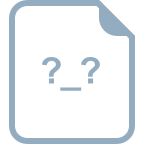
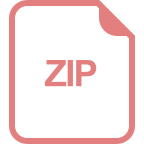
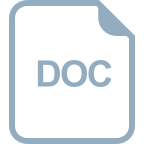
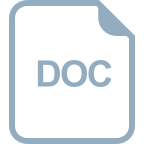


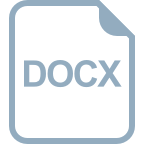
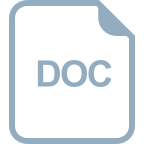