求解全排列问题,给定n个互不相同的元素,实现求解全排列的函数,用c语言
时间: 2024-10-07 14:08:23 浏览: 27
在C语言中,求解n个互不相同的元素的全排列问题,可以使用递归的方式来实现。这里是一个基本的递归函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个数组存储当前的排列
#define ARRAY_SIZE 10
int permutation[ARRAY_SIZE];
// 辅助函数用于生成下一个排列
void generatePermutation(int n, int index) {
if (index == n) { // 如果已经生成到第n个位置,结束递归
for (int i = 0; i < n; i++) {
printf("%d ", permutation[i]);
}
printf("\n");
} else {
for (int i = index; i < n; i++) { // 将剩余数填入当前位置
permutation[index] = i;
generatePermutation(n, index + 1); // 继续生成下一个位置的排列
}
}
}
// 主函数调用辅助函数开始全排列过程
void findPermutations(int n) {
permutation[0] = 0;
generatePermutation(n, 1);
}
int main() {
int numElements;
printf("Enter the number of elements: ");
scanf("%d", &numElements);
if (numElements <= 0 || numElements > ARRAY_SIZE) {
printf("Invalid input! Please enter a positive number less than or equal to %d.\n", ARRAY_SIZE);
return 1;
}
findPermutations(numElements);
return 0;
}
```
这个程序首先从第一个元素开始,然后将剩余元素依次插入到当前元素的位置上,形成一个新的排列。当所有元素都填完后,就得到一个完整的排列。注意,这种方法的时间复杂度是O(n!),因为有n!种可能的排列。
阅读全文
相关推荐
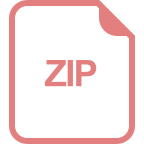
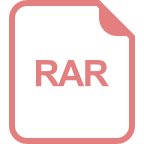
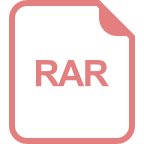


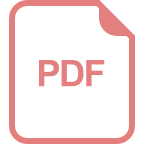
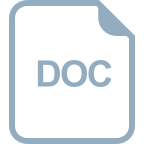
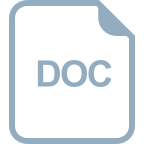
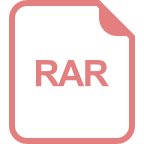
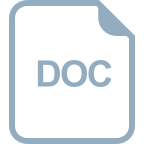
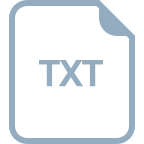
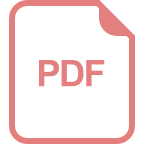
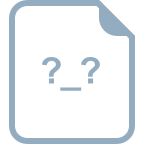
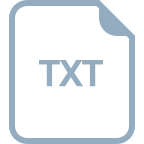
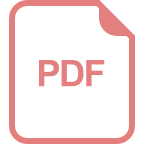
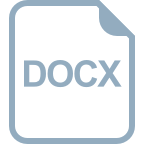
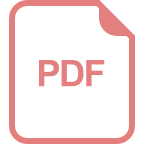