用c#查询sql显示到datagridview中,将选中行生成条码,扫码枪扫描二维码能弹出窗体显示选中行的信息
时间: 2024-09-14 11:13:57 浏览: 45
在C#中,为了实现这个功能,你需要做以下几个步骤:
1. **连接数据库并执行查询**:
首先,使用`SqlConnection`对象建立与SQL Server的连接,然后编写一个SQL查询,例如使用`SqlCommand`执行查询并将结果填充到`DataTable`或`DataSet`中。
```csharp
string connectionString = "your_connection_string";
string sqlQuery = "SELECT * FROM your_table";
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(sqlQuery, connection);
SqlDataAdapter adapter = new SqlDataAdapter(command);
DataTable dataTable = new DataTable();
adapter.Fill(dataTable);
dataGridView.DataSource = dataTable;
}
```
2. **数据绑定至DataGridView**:
将查询结果绑定到DataGridView上,以便用户能看到数据。
```csharp
dataGridView.Columns.Add("Column1", "Column 1");
dataGridView.Columns.Add("Column2", "Column 2"); // 根据实际表结构添加列
// 确保数据网格显示所有列
dataGridView.AutoGenerateColumns = true;
```
3. **选中行事件处理**:
通过设置`dataGridView.SelectionChanged`事件,在用户选择某一行时触发。这时可以获取所选行的数据,并准备生成条形码。
```csharp
dataGridView.getSelectionModel().SelectedIndexChanged += DataGridView_SelectedIndexChanged;
private void DataGridView_SelectedIndexChanged(object sender, EventArgs e)
{
DataGridViewRow selectedRow = dataGridView.CurrentRow;
if (selectedRow != null)
{
// 获取当前行数据
object dataValue = selectedRow.Cells["YourColumn"].Value; // 更改为实际的列名
GenerateBarcode(dataValue); // 生成条码
}
}
```
4. **生成条码**:
使用第三方库如`ZBarNet`、`CodeFirst Barcode Generator`等来创建二维码,并将其显示在一个新的窗口或模态对话框中供扫码枪扫描。
```csharp
private void GenerateBarcode(object rowData)
{
// 创建二维码图片
BarcodeGenerator barcodeGen = new BarcodeGenerator(BarcodeTypes.QRCode, Convert.ToString(rowData));
Image barcodeImage = barcodeGen.GenerateImage();
// 显示二维码
using (Form form = new Form())
{
form.Text = $"QR Code for Row {selectedRow.Index}";
PictureBox pictureBox = new PictureBox();
pictureBox.Image = barcodeImage;
form.Controls.Add(pictureBox);
form.ShowDialog(); // 弹出窗体展示二维码
}
}
阅读全文
相关推荐




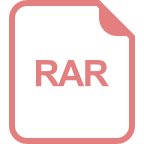













