使用python1、访问桌面上的“荷塘月色.txt”。 2、要求将所有字符按照频次从高到低排序,字符包括中文、标点、英文等符号,但不包含空格和回车。 3、字符与频次之间采用英文冒号″:"分隔,各字符之间釆用英文逗号″,″分隔。4、输出成文档到桌面
时间: 2024-09-25 10:19:09 浏览: 34
要完成这个任务,我们需要使用Python的一些内置库,如`os`, `openpyxl`(用于读取文本文件)以及`collections`(用于计数字符频率)。以下是如何逐步实现这些步骤:
1. 首先,我们需要获取桌面路径。在Windows中,桌面上的路径可能不是标准的,通常可以通过环境变量 `%USERPROFILE%` 来表示。但在实际操作中,由于安全原因,直接使用环境变量可能会有问题,我们可以创建一个临时文件夹存储文件。
```python
import os
# 创建一个临时目录来保存文件,假设用户桌面路径为'c:\Users\用户名\Desktop'
temp_dir = os.path.join(os.environ['USERPROFILE'], 'Desktop', 'temp')
if not os.path.exists(temp_dir):
os.makedirs(temp_dir)
# 将文本文件移动到临时目录
file_path = os.path.join(temp_dir, "荷塘月色.txt")
```
2. 接下来,我们打开文本文件并逐行读取内容,然后统计字符出现的次数。
```python
with open(file_path, 'r', encoding='utf-8') as f:
text_content = f.read()
# 过滤掉空格和换行符
filtered_text = ''.join(c for c in text_content if c.isalnum() or c.isspace())
# 使用Counter计算字符频率
from collections import Counter
char_freq = Counter(filtered_text)
```
3. 对于输出,我们将字符和对应的频次按照需求格式化。这里我们使用字符串格式化的方法。
```python
sorted_chars = sorted(char_freq.items(), key=lambda x: x[1], reverse=True) # 按照频次降序排列
output_string = ''
for char, freq in sorted_chars:
output_string += f"{char}: {freq}, " # 英文冒号和逗号分隔
# 去掉末尾多余的逗号
output_string = output_string[:-2] + '\n'
# 再次写入一个新的文本文件
output_file_path = os.path.join(temp_dir, "character_frequency.txt")
with open(output_file_path, 'w', encoding='utf-8') as f:
f.write(output_string)
```
4. 最后,我们将结果保存到桌面上的指定位置。
阅读全文
相关推荐
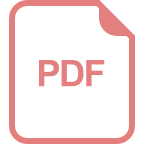
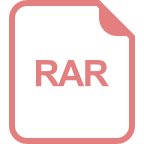
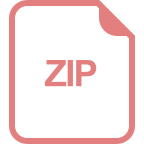


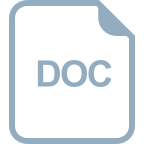










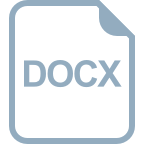