查找顺序表中的最大值,并将最大值移动到顺序表的末尾。其余元素线性关系不变。c语言
时间: 2024-10-19 10:04:31 浏览: 26
要在C语言中实现这个功能,可以使用双指针方法。下面是一种可能的解决方案:
```c
#include <stdio.h>
void find_max_and_push(int* array, int size) {
int max = array[0]; // 假设第一个元素是最大的
int i;
for (i = 1; i < size; i++) {
if (array[i] > max) {
max = array[i]; // 如果当前元素大于当前最大值,则更新最大值
}
}
// 找到最大值后,将其放到数组的末尾
for (i = size - 1; i >= 0; i--) {
if (array[i] == max) { // 如果当前元素等于最大值
array[i + 1] = array[i]; // 将最大值移动到数组的末尾
break;
}
}
}
int main() {
int array[] = {1, 5, 3, 7, 2, 6}; // 假设这是你的顺序表
int size = sizeof(array) / sizeof(array[0]); // 获取顺序表的长度
find_max_and_push(array, size); // 查找并移动最大值到数组的末尾
for (int i = 0; i < size; i++) {
printf("%d ", array[i]); // 打印数组,可以看到最大值已经移动到了末尾
}
return 0;
}
```
这段代码首先定义了一个函数`find_max_and_push`,它接受一个整数数组和数组的大小作为输入。这个函数通过遍历数组找到最大值,并将其移动到数组的末尾。在主函数`main`中,我们创建了一个示例数组并调用了`find_max_and_push`函数。最后,我们打印出数组的内容,可以看到最大值已经被移动到了数组的末尾。
阅读全文
相关推荐
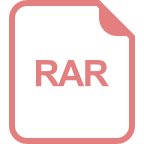
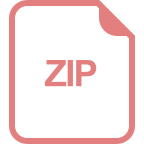
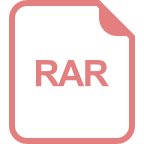
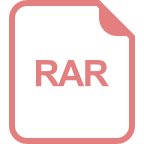
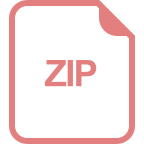
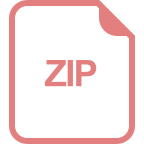
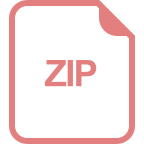
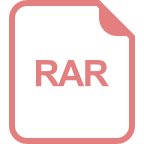
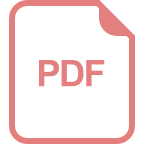
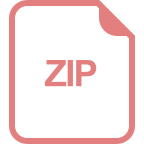
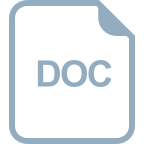
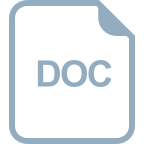
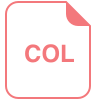
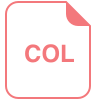
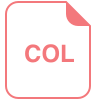


