使用C#写一段保存文本内容到CSV文件的代码
时间: 2024-11-16 22:27:26 浏览: 6
在C#中,你可以使用`System.IO`和`CsvHelper`库来方便地将数据保存到CSV文件。首先,你需要安装`CsvHelper`库,可以使用NuGet包管理器添加。下面是一个简单的例子:
```csharp
using System;
using System.IO;
using CsvHelper;
class Program
{
static void Main(string[] args)
{
// 假设我们有学生数据模型
class Student
{
public string Name { get; set; }
public int Age { get; set; }
}
// 创建一些示例学生对象
var students = new List<Student>
{
new Student { Name = "Alice", Age = 20 },
new Student { Name = "Bob", Age = 22 },
new Student { Name = "Charlie", Age = 19 }
};
// 定义CSV文件路径
string filePath = "students.csv";
// 创建CSV writer
using (var writer = new StreamWriter(filePath))
using (var csv = new CsvWriter(writer, CultureInfo.InvariantCulture))
{
// 写入表头
csv.WriteRecords(students.Select(x => x.GetType().GetProperties()));
// 写入数据
csv.WriteRecords(students);
}
Console.WriteLine($"Data saved to {filePath}");
}
}
```
在这个例子中,我们首先创建了一个包含学生属性的对象列表,然后使用`CsvWriter`逐行写入数据。注意这里先写表头,然后写数据,因为`WriteRecords`方法会自动检测是否需要写入表头。
阅读全文
相关推荐
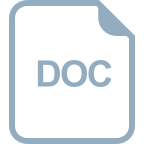
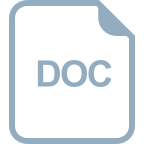
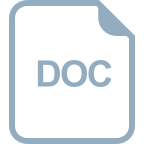
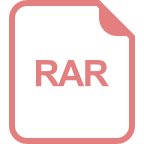
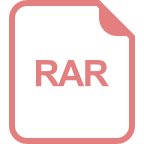
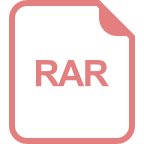
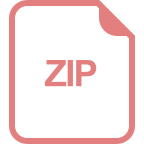
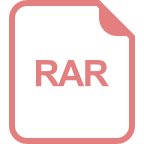
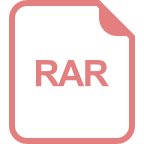
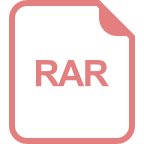
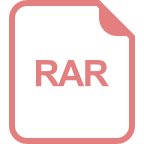
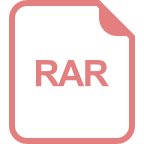
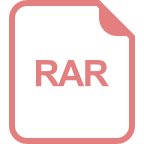
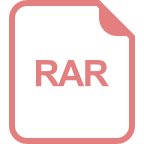
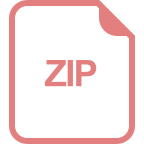
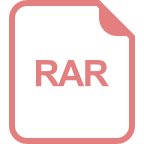
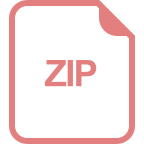
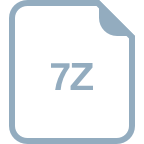
