OpenCV如何正确处理并发图片读取?
时间: 2024-10-06 18:04:02 浏览: 22
在OpenCV中正确处理并发图片读取通常涉及以下几个步骤:
1. **线程安全**: 使用`cv2.VideoCapture`时,虽然它内部已经做了线程同步,但为了保险起见,最好在每个读取操作前后加上适当的锁,比如使用Python的`threading.Lock`。
```python
import cv2
import threading
lock = threading.Lock()
def read_image(file_path):
lock.acquire()
try:
cap = cv2.VideoCapture(file_path)
_, frame = cap.read()
# ...此处处理帧数据...
finally:
cap.release()
lock.release()
```
2. **多线程池**: 如果有大量的图片需要读取,可以考虑使用多线程池(如`concurrent.futures.ThreadPoolExecutor`),将任务分发到多个工作线程上,提高效率。
3. **队列机制**: 创建一个队列,线程从队列中取出任务,而不是直接访问文件。这样能保证图片的顺序和避免阻塞主线程。
```python
from queue import Queue
import threading
queue = Queue()
# ...添加图片到队列...
def worker():
while True:
file_path = queue.get()
if not file_path:
break
# 在这里处理图片
...
threads = [threading.Thread(target=worker) for _ in range(thread_count)]
for thread in threads:
thread.start()
for _ in range(len(threads)):
queue.put(None) # 指示线程结束
threads.pop().join()
```
这样可以更好地控制并行度,同时保证了资源的有效利用。
阅读全文
相关推荐
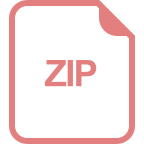
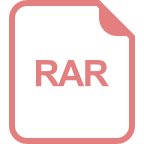
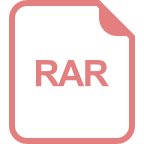
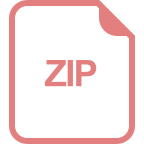
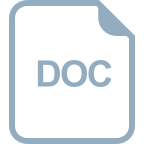
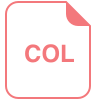
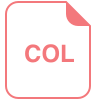
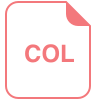
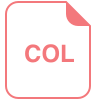
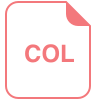
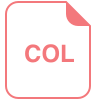
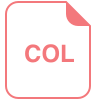
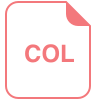
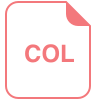
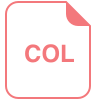



