java中queue 存储坐标
时间: 2024-10-18 14:17:51 浏览: 37
在Java中,如果你需要存储坐标数据并使用Queue(队列)的数据结构,可以考虑使用`PriorityQueue`、`LinkedList`或`ArrayDeque`,因为它们都是线程安全的。例如,你可以创建一个自定义的`Coordinate`类来封装坐标的X和Y值:
```java
import java.util.*;
class Coordinate {
int x;
int y;
public Coordinate(int x, int y) {
this.x = x;
this.y = y;
}
// 可以添加getter和setter方法以及equals()和hashCode()方法,用于比较和哈希
}
public class Main {
Queue<Coordinate> queue = new PriorityQueue<>((a, b) -> a.getX() - b.getX()); // 按X坐标排序
void enqueue(Coordinate coord) {
queue.offer(coord);
}
Coordinate dequeue() {
if (queue.isEmpty()) {
return null; // 或者抛出异常,取决于你的处理策略
}
return queue.poll();
}
public static void main(String[] args) {
Main example = new Main();
example.enqueue(new Coordinate(0, 0)); // 入队(0, 0)
example.enqueue(new Coordinate(5, 3)); // 入队(5, 3)
while (!example.queue.isEmpty()) { // 遍历队列
System.out.println(example.dequeue());
}
}
}
```
阅读全文
相关推荐




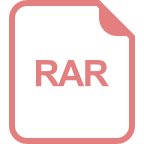

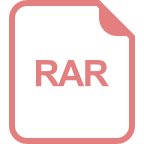

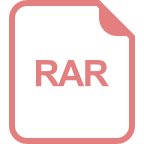







