:图像编辑和游戏开发中的实际应用:Java种子填充算法的应用案例解析
发布时间: 2024-08-28 10:29:45 阅读量: 18 订阅数: 24 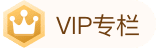
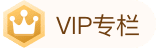
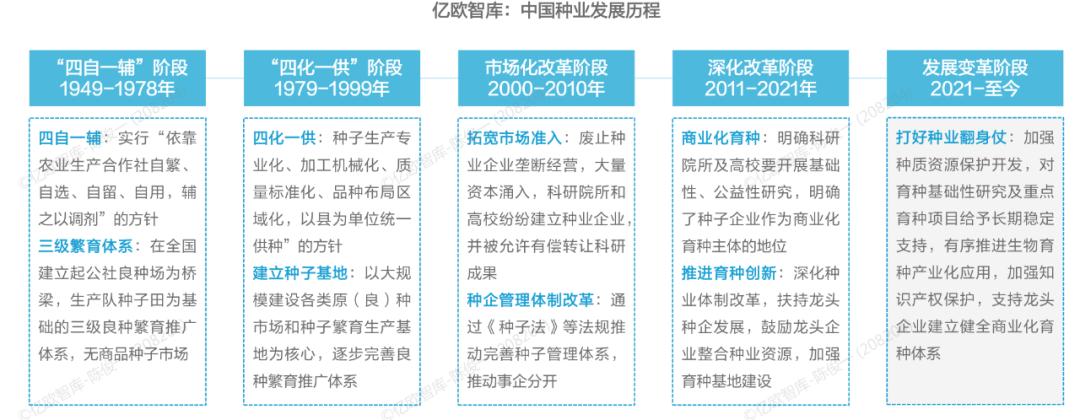
# 1. Java种子填充算法概述**
种子填充算法是一种图像处理技术,用于填充图像中指定区域内的像素,使其与相邻的像素具有相同的颜色或值。在Java中,种子填充算法通常通过递归或迭代的方式实现,通过遍历相邻像素并检查其颜色或值是否与种子像素相同来填充区域。
种子填充算法在图像编辑、游戏开发和其他需要填充封闭区域的应用中有着广泛的应用。它可以用于填充选定的区域、创建渐变效果或生成随机纹理。
# 2. Java种子填充算法的理论基础
### 2.1 图像处理中的种子填充算法
**定义:**
种子填充算法是一种图像处理技术,用于填充图像中指定区域的颜色或值。它使用一个种子点作为起点,并递归地填充与种子点相邻且具有相同或相似颜色的像素。
**原理:**
种子填充算法的原理如下:
1. 从图像中选择一个种子点。
2. 检查种子点周围的像素。
3. 如果像素与种子点的颜色相同或相似,则将其填充为种子点的颜色。
4. 递归地检查填充后的像素周围的像素,重复步骤 2-3,直到所有相邻的相同颜色像素都被填充。
### 2.2 Java种子填充算法的原理和实现
**Java实现:**
Java中可以使用以下算法实现种子填充:
```java
public static void seedFill(int[][] image, int x, int y, int newColor) {
if (image[x][y] == newColor) {
return;
}
int oldColor = image[x][y];
image[x][y] = newColor;
if (x > 0 && image[x - 1][y] == oldColor) {
seedFill(image, x - 1, y, newColor);
}
if (x < image.length - 1 && image[x + 1][y] == oldColor) {
seedFill(image, x + 1, y, newColor);
}
if (y > 0 && image[x][y - 1] == oldColor) {
seedFill(image, x, y - 1, newColor);
}
if (y < image[0].length - 1 && image[x][y + 1] == oldColor) {
seedFill(image, x, y + 1, newColor);
}
}
```
**参数说明:**
* `image`:要填充的图像(二维数组)
* `x`:种子点的 x 坐标
* `y`:种子点的 y 坐标
* `newColor`:填充的颜色
**逻辑分析:**
1. 首先,检查种子点是否已经填充为 `newColor`,如果是,则返回。
2. 将种子点的颜色保存到 `oldColor` 变量中。
3. 将种子点填充为 `newColor`。
4. 检查种子点周围的四个方向(左、右、上、下)的像素是否与 `oldColor` 相同。
5. 如果相邻像素与 `oldColor` 相同,则递归地调用 `seedFill` 方法,以填充该像素。
**代码块执行逻辑说明:**
```java
// 检查种子点是否已经填充为 newColor
if (image[x][y] == newColor) {
return;
}
```
如果种子点已经填充为 `newColor`,则算法直接返回,无需进一步填充。
```java
// 将种子点的颜色保存到 oldColor 变量中
int oldColor = image[x][y];
```
将种子点的原始颜色保存到 `oldColor` 变量中,以便在递归填充时进行比较。
```java
// 将种子点填充为 newColor
image[x][y] = newColor;
```
将种子点填充为 `newColor`,作为填充的起点。
```java
// 检查种子点周围的四个方向的像素是否与 oldColor 相同
if (x > 0 && image[x - 1][y] == oldColor) {
seedFill(image, x - 1, y, newColor);
}
if (x < image.length - 1 && image[x + 1][y] == oldColor) {
seedFill(image, x + 1, y, newColor);
}
if (y > 0 && image[x][y - 1] == oldColor) {
seedFill(image, x, y - 1, newColor);
}
if (y < image[0].length - 1 && image[x][y + 1] == oldColor) {
seedFill(image, x, y + 1, newColor);
}
```
检查种子点周围的四个方向(左、右、上、下)的像素是否与 `oldColor` 相同。如果相邻像素与 `oldColor` 相同,则递归地调用 `seedFill` 方法,以填充该像素。
# 3. Java种子填充算法的实践应用**
### 3.1 图像编辑中的种子填充
#### 3.1.1 图像填充的原理和步骤
图像填充算法是一种计算机图形学技术,用于将图像中的封闭区域填充为指定的颜色或图案。种子填充算法是图像填充算法中最常用的方法之一,其原理如下:
1. **选择种子点:**用户在图像中选择一个点作为种子点,该点位于要填充区域的内部。
2. **检查邻域:**从种子点开始,算法检查其相邻的像素点。如果相邻像素点与种子点颜色相同,则将其标记为待填充像素。
3. **递归填充:**对所有标记为待填充的像素点重复步骤 2,直到填充整个区域。
#### 3.1.2 Java实现的图像填充示例
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
public class ImageFiller {
public static void fill(BufferedImage image, int x, int y, Color fillColor) {
int width = image.getWidth();
int height = image.getHeight();
// 检查边界条件
if (x < 0 || x >= width || y < 0 || y >= height) {
return;
}
// 获取种子点的颜色
Color seedColor = new Color(image.getRGB(x, y));
// 创建待填充像素队列
Queue<Point> queue = new LinkedList<>();
queue.add(new Point(x, y));
// 循环填充
while (!queue.isEmpty()) {
Point current = queue.poll();
// 检查当前像素是否与种子点颜色相同
if (image.getRGB(current.x, current.y) != seedColor.getRGB()) {
continue;
}
// 填充当前像素
image.setRGB(current.x, current.y, fillColor.getRGB());
// 将相邻像素添加到队列中
if (current.x > 0) {
queue.add(new Point(current.x - 1, current.y));
}
if (current.x < width - 1) {
queue.add(new Point(current.x + 1, current.y));
}
if (current.y > 0) {
queue.add(new Point(current.x, current.y - 1));
}
if (current.y < height - 1) {
qu
```
0
0
相关推荐
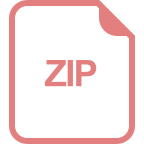
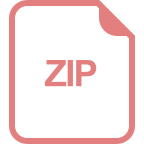
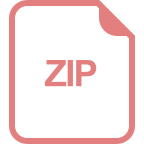
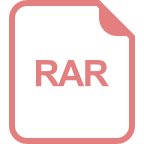
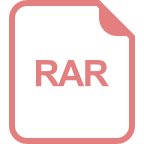
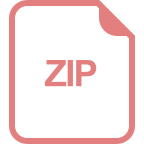
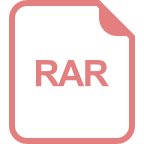
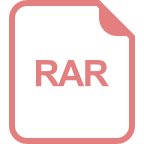