,Java种子填充算法性能优化:提升填充效率的6大秘诀
发布时间: 2024-08-28 10:08:29 阅读量: 8 订阅数: 11 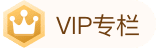
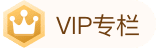
# 1. Java种子填充算法概述
种子填充算法是一种广泛用于计算机图形学和图像处理的算法,用于填充封闭区域。其原理是:从一个种子点开始,向周围扩展,直到遇到边界或已填充区域。
种子填充算法具有以下特点:
* **简单易懂:**算法原理简单,易于理解和实现。
* **高效快速:**算法时间复杂度通常为 O(n),其中 n 为填充区域的像素数量。
* **广泛适用:**算法可用于填充任意形状的封闭区域,包括凸多边形和凹多边形。
# 2. 种子填充算法的理论基础
种子填充算法是一种用于填充封闭区域的计算机图形算法。它通过从种子点开始,逐步填充与种子点相邻的像素,直到遇到边界或填充区域外的像素为止。
### 2.1 扫描线填充算法
**2.1.1 算法原理**
扫描线填充算法是一种基于扫描线的种子填充算法。它从种子点开始,沿水平方向扫描图像,并填充与种子点相邻的像素。当扫描线遇到边界或填充区域外的像素时,扫描线停止填充并继续扫描下一行。
**2.1.2 算法优缺点**
* 优点:
* 简单易于实现
* 填充速度快
* 缺点:
* 对于复杂形状的区域,可能产生阶梯状伪影
* 对于大面积区域,填充效率较低
### 2.2 边界填充算法
**2.2.1 算法原理**
边界填充算法是一种基于边界的种子填充算法。它从种子点开始,沿着边界扫描图像,并填充与边界相邻的像素。当扫描边界遇到边界或填充区域外的像素时,扫描边界停止填充并继续扫描下一个边界。
**2.2.2 算法优缺点**
* 优点:
* 对于复杂形状的区域,填充效果较好
* 对于大面积区域,填充效率较高
* 缺点:
* 实现复杂度较高
* 填充速度较慢
**代码示例:**
```java
// 扫描线填充算法
public void scanLineFill(int x, int y, int fillColor) {
// 获取图像尺寸
int width = image.getWidth();
int height = image.getHeight();
// 检查边界
if (x < 0 || x >= width || y < 0 || y >= height) {
return;
}
// 获取种子点颜色
int seedColor = image.getRGB(x, y);
// 扫描线填充
for (int i = y; i < height; i++) {
// 填充扫描线
for (int j = x; j < width; j++) {
if (image.getRGB(j, i) == seedColor) {
image.setRGB(j, i, fillColor);
}
}
}
}
// 边界填充算法
public void boundaryFill(int x, int y, int fillColor) {
// 获取图像尺寸
int width = image.getWidth();
int height = image.getHeight();
// 检查边界
if (x < 0 || x >= width || y < 0 || y >= height) {
return;
}
// 获取种子点颜色
int seedColor = image.getRGB(x, y);
// 边界填充
if (image.getRGB(x, y) != fillColor) {
image.setRGB(x, y, fillColor);
boundaryFill(x + 1, y, fillColor);
boundaryFill(x - 1, y, fillColor);
boundaryFill(x, y + 1, fillColor);
boundaryFill(x, y - 1, fillColor);
}
}
```
**代码逻辑分析:**
* `scanLineFill()` 函数从种子点开始,沿水平方向扫描图像,并填充与种子点相邻的像素。
* `boundaryFill()` 函数从种子点开始,沿着边界扫描图像,并填充与边界相邻的像素。
* 两个函数都使用递归来遍历图像,并填充与种子点或边界相邻的像素。
**参数说明:**
* `x`:种子点的横坐标
0
0
相关推荐
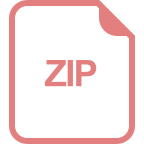
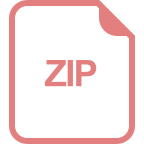
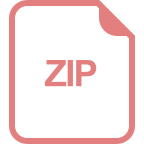





