python使用迭代器实现斐波那契数列
时间: 2023-11-24 16:37:32 浏览: 96
要使用迭代器来实现斐波那契数列,可以定义一个类,并在该类中添加`__iter__`方法和`__next__`方法。首先,设置初始值a为0,b为1。在`__next__`方法中,返回当前的a的值,并更新a和b的值。同时,通过添加一个计数器来控制数列的长度。当计数器达到指定的长度时,抛出StopIteration异常来终止迭代。最后,创建该类的实例,并使用`list()`函数将迭代结果转换为列表形式输出。
以下是实现斐波那契数列的迭代器的示例代码:
```python
class Fibonacci:
def __init__(self, length):
self.length = length
self.a = 0
self.b = 1
self.counter = 0
def __iter__(self):
return self
def __next__(self):
if self.counter >= self.length:
raise StopIteration
fibonacci_number = self.a
self.a, self.b = self.b, self.a + self.b
self.counter += 1
return fibonacci_number
fibonacci_iterator = Fibonacci(10)
fibonacci_list = list(fibonacci_iterator)
print(fibonacci_list)
```
运行结果为:[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
阅读全文
相关推荐
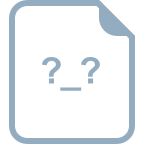
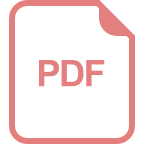
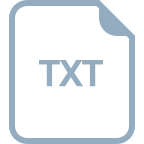
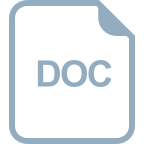

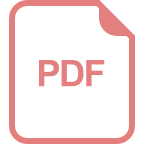
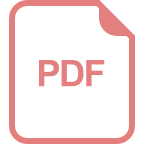
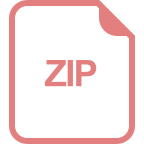
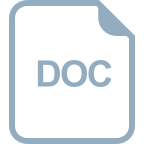
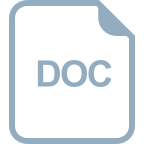
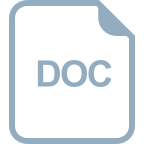
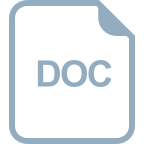
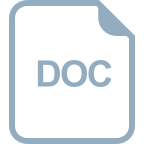
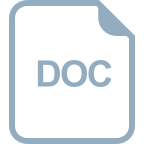



