在c++中,调用其他源文件进行递归阶乘
时间: 2024-11-22 17:46:56 浏览: 17
在 C++ 中,递归阶乘是一个经典的例子,用于演示函数在同个源文件或另一个源文件之间的相互调用。假设我们想计算一个数 n 的阶乘,可以将递归函数拆分成两个部分:一个主程序负责调用函数,另一个单独的函数实现递归过程。
首先,在头文件(如 `factorial.h`)中定义一个返回整数的模板函数,以便在需要的地方重用:
```cpp
// factorial.h
#ifndef FACTORIAL_H
#define FACTORIAL_H
#include <iostream>
template <typename T>
T factorial(T num);
#endif // FACTORIAL_H
```
然后在实现文件(如 `factorial.cpp`)中编写递归函数的具体实现:
```cpp
// factorial.cpp
#include "factorial.h"
template <typename T>
T factorial(T num) {
if (num == 0 || num == 1) {
return 1;
} else {
return num * factorial(num - 1);
}
}
```
要在主程序中调用这个函数,比如在 `main.cpp` 文件里:
```cpp
// main.cpp
#include "factorial.h"
#include <iostream>
int main() {
int number;
std::cout << "Enter a non-negative integer for its factorial: ";
std::cin >> number;
long long result = factorial<int>(number); // 使用long long防止溢出
std::cout << "The factorial of " << number << " is: " << result << std::endl;
return 0;
}
```
现在,你可以分别编译这两个文件(`g++ main.cpp factorial.cpp -o program`),运行 `program` 来测试递归阶乘功能。记得处理好大数溢出的问题,这里通过使用 `long long` 类型来缓解这个问题。
阅读全文
相关推荐
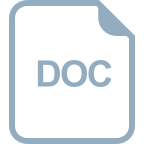
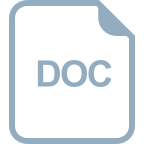
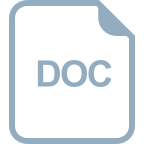

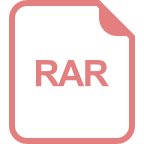
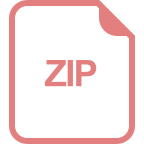
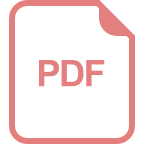
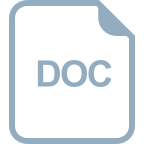
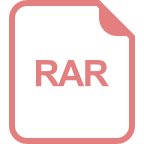
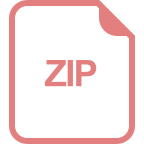
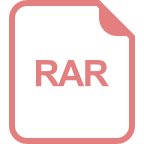
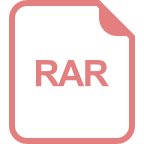
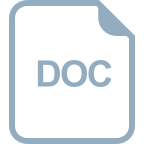
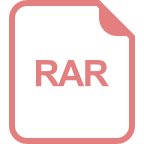
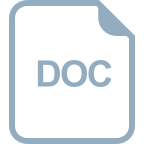
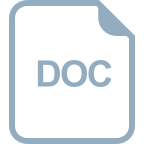
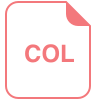
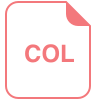
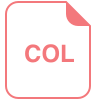