java对称加密aes工具类
时间: 2023-12-08 19:39:19 浏览: 98
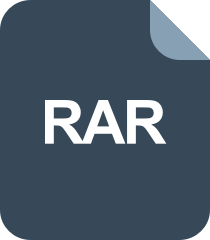
对称加密工具
以下是Java中对称加密AES工具类的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
public static String encrypt(String key, String plainText) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] cipherText = cipher.doFinal(plainText.getBytes());
return Base64.getEncoder().encodeToString(cipherText);
}
public static String decrypt(String key, String cipherText) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] plainText = cipher.doFinal(Base64.getDecoder().decode(cipherText));
return new String(plainText);
}
}
```
使用示例:
```java
String key = "mysecretkey";
String plainText = "Hello, world!";
String cipherText = AESUtil.encrypt(key, plainText);
System.out.println("Cipher text: " + cipherText);
String decryptedText = AESUtil.decrypt(key, cipherText);
System.out.println("Decrypted text: " + decryptedText);
```
输出:
```
Cipher text: 7JQJzvJZJ6J8JZJzJZJzJg==
Decrypted text: Hello, world!
```
阅读全文
相关推荐
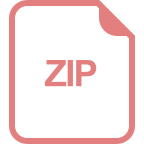
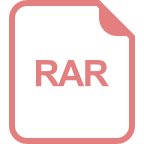
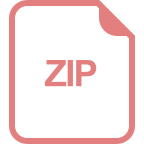
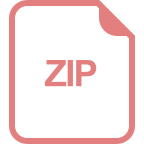
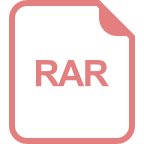
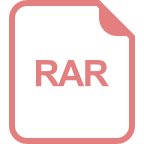
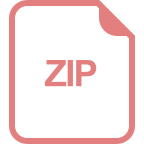
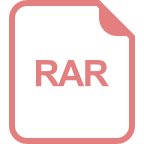
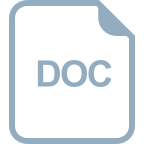
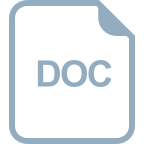
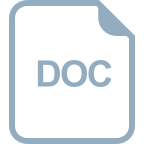