写一段关于顺序表创建的C语言代码
时间: 2024-10-03 09:00:52 浏览: 29
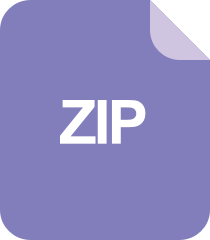
数据结构2-线性表之顺序储存(顺序表)C语言版
当然,这里有一段简单的C语言代码,用于创建一个顺序表(单链表),并演示了如何插入节点:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构体
typedef struct Node {
int value; // 节点值
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建顺序表的函数
Node* createSequentialList(int capacity) {
if (capacity <= 0) {
printf("Error: Capacity must be positive.\n");
return NULL;
}
Node* list = (Node*) malloc(capacity * sizeof(Node)); // 动态分配内存
list[0].value = -1; // 初始化第一个空节点
list[0].next = NULL;
return list;
}
// 在顺序表末尾插入节点的函数
void insertAtEnd(Node** head, int value) {
Node* newNode = (Node*) malloc(sizeof(Node));
newNode->value = value;
newNode->next = NULL;
if (*head == NULL) { // 列表为空,直接赋值
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印顺序表
void printSequentialList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
} else {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->value);
temp = temp->next;
}
printf("NULL\n");
}
}
int main() {
int capacity = 5;
Node* sequentialList = createSequentialList(capacity);
// 插入一些元素
insertAtEnd(&sequentialList, 10);
insertAtEnd(&sequentialList, 20);
insertAtEnd(&sequentialList, 30);
// 打印顺序表
printSequentialList(sequentialList);
// 清理内存
free(sequentialList);
return 0;
}
```
这段代码首先创建了一个顺序表,然后通过`insertAtEnd`函数在末尾插入元素,最后打印整个列表。记得在程序结束时释放动态分配的内存,防止内存泄漏。
阅读全文
相关推荐
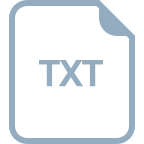
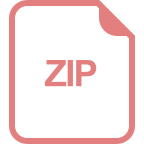
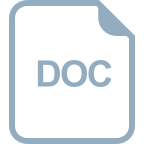




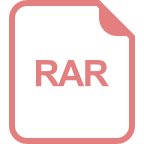
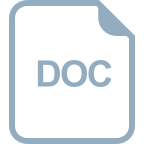








