用C语言写一段代码:设计一个算法,删除顺序表中值为x的所有节点。
时间: 2024-09-27 16:08:43 浏览: 48
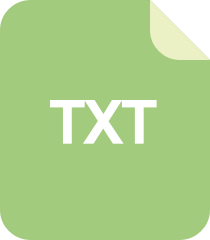
十大滤波算法程序大全

在C语言中,你可以使用循环遍历顺序表(数组或链表),并检查每个元素是否等于目标值x。如果找到等于x的元素,就将其移除。这里是一个简单的例子,假设我们有一个单向链表结构:
```c
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 删除链表中值为x的节点
void deleteNodes(Node** head, int x) {
// 避免头部为空的情况
if (*head == NULL && (*head)->data == x) {
*head = NULL;
return;
}
Node* current = *head;
Node* prev = NULL;
// 循环查找并删除
while (current != NULL) {
if (current->data == x) {
// 如果当前节点就是要删除的
if (prev == NULL) { // 头部节点
*head = current->next;
} else {
prev->next = current->next;
}
free(current); // 释放内存
current = *head; // 更新当前节点到下一个
} else {
prev = current; // 没有找到,继续前进
}
}
}
// 示例:创建链表并删除所有值为x的节点
Node* createList(int size, int x) {
// ... 创建链表代码 ...
for (int i = 0; i < size; ++i) {
if (i == x) {
deleteNodes(&head, x);
} else {
// 添加节点...
}
}
return head;
}
```
阅读全文
相关推荐
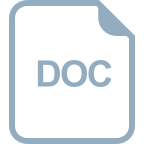
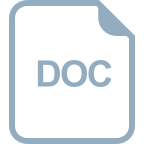













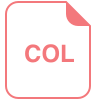
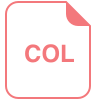
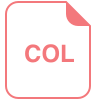