websocket Php
时间: 2023-12-23 22:04:02 浏览: 84
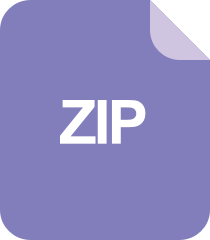
php-websockets
以下是一个使用PHP实现WebSocket实时消息推送的示例:
```php
<?php
class SocketService {
private $address = '0.0.0.0';
private $port = 8080;
private $socket;
public function __construct() {
$this->createSocket();
$this->bindSocket();
$this->listenForConnections();
}
private function createSocket() {
$this->socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
if ($this->socket === false) {
echo "Failed to create socket: " . socket_strerror(socket_last_error()) . "\n";
exit(1);
}
}
private function bindSocket() {
if (!socket_bind($this->socket, $this->address, $this->port)) {
echo "Failed to bind socket: " . socket_strerror(socket_last_error($this->socket)) . "\n";
exit(1);
}
}
private function listenForConnections() {
if (!socket_listen($this->socket)) {
echo "Failed to listen for connections: " . socket_strerror(socket_last_error($this->socket)) . "\n";
exit(1);
}
echo "Server started. Listening for connections...\n";
while (true) {
$clientSocket = socket_accept($this->socket);
if ($clientSocket === false) {
echo "Failed to accept connection: " . socket_strerror(socket_last_error($this->socket)) . "\n";
continue;
}
$this->handleClient($clientSocket);
}
}
private function handleClient($clientSocket) {
$request = socket_read($clientSocket, 1024);
$response = "HTTP/1.1 101 Switching Protocols\r\n" .
"Upgrade: websocket\r\n" .
"Connection: Upgrade\r\n" .
"Sec-WebSocket-Accept: " . $this->generateAcceptKey($request) . "\r\n\r\n";
socket_write($clientSocket, $response, strlen($response));
socket_close($clientSocket);
}
private function generateAcceptKey($request) {
$key = '';
if (preg_match("/Sec-WebSocket-Key: (.*)\r\n/", $request, $matches)) {
$key = $matches[1];
}
return base64_encode(sha1($key . '258EAFA5-E914-47DA-95CA-C5AB0DC85B11', true));
}
}
$socketService = new SocketService();
```
这个示例创建了一个WebSocket服务器,监听在本地的8080端口。当有客户端连接时,服务器会发送一个升级协议的响应,告诉客户端即将升级为WebSocket协议。这个示例只是一个简单的演示,实际应用中可能需要添加更多的功能和处理逻辑。
阅读全文
相关推荐
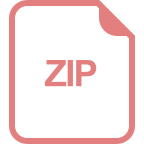
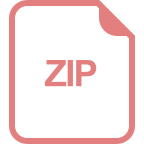
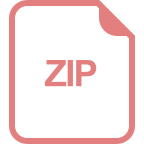
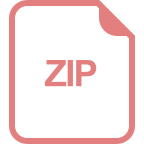

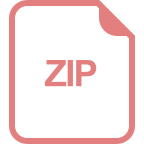
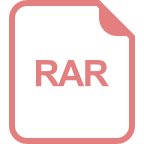







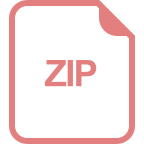
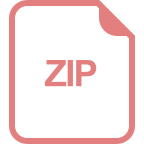